Introduction
This is a simple Android ordering system for Healthware, a healthcare company offering products like hair care, vitamins, skincare, and more. With a user-friendly UI built in Android Studio and powered by Firebase, it’s all about making shopping easy and convenient.
Application Features
- User login and registration
- View list of products with details
- Add items to cart
- Calculate the total price of the items added to the cart
- Check out
- View Order History
- Edit profile
Print Screen and Code
1. Login Page
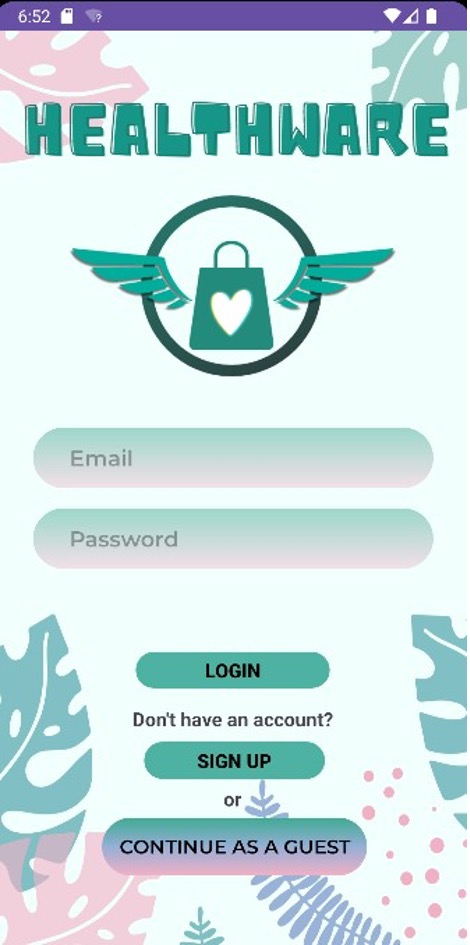
1.1 Handling Login for Users without an Account (Register Page)
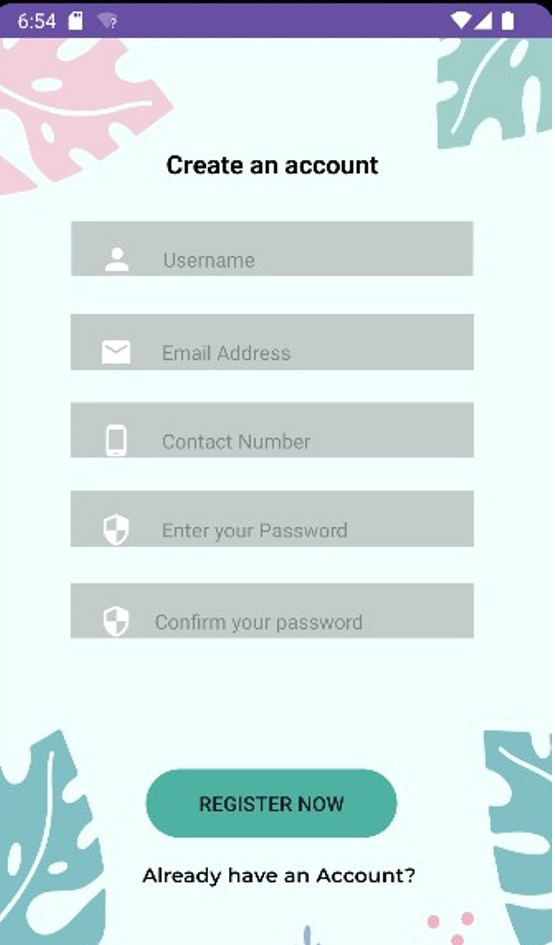
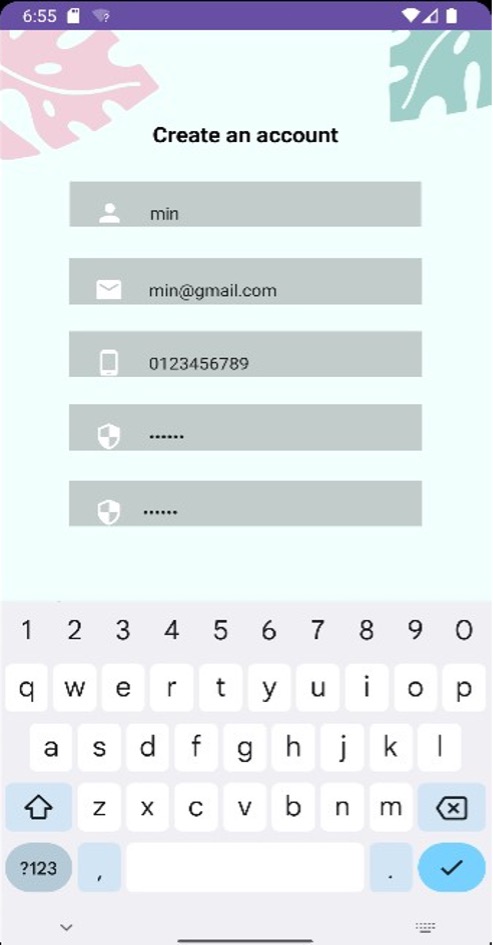
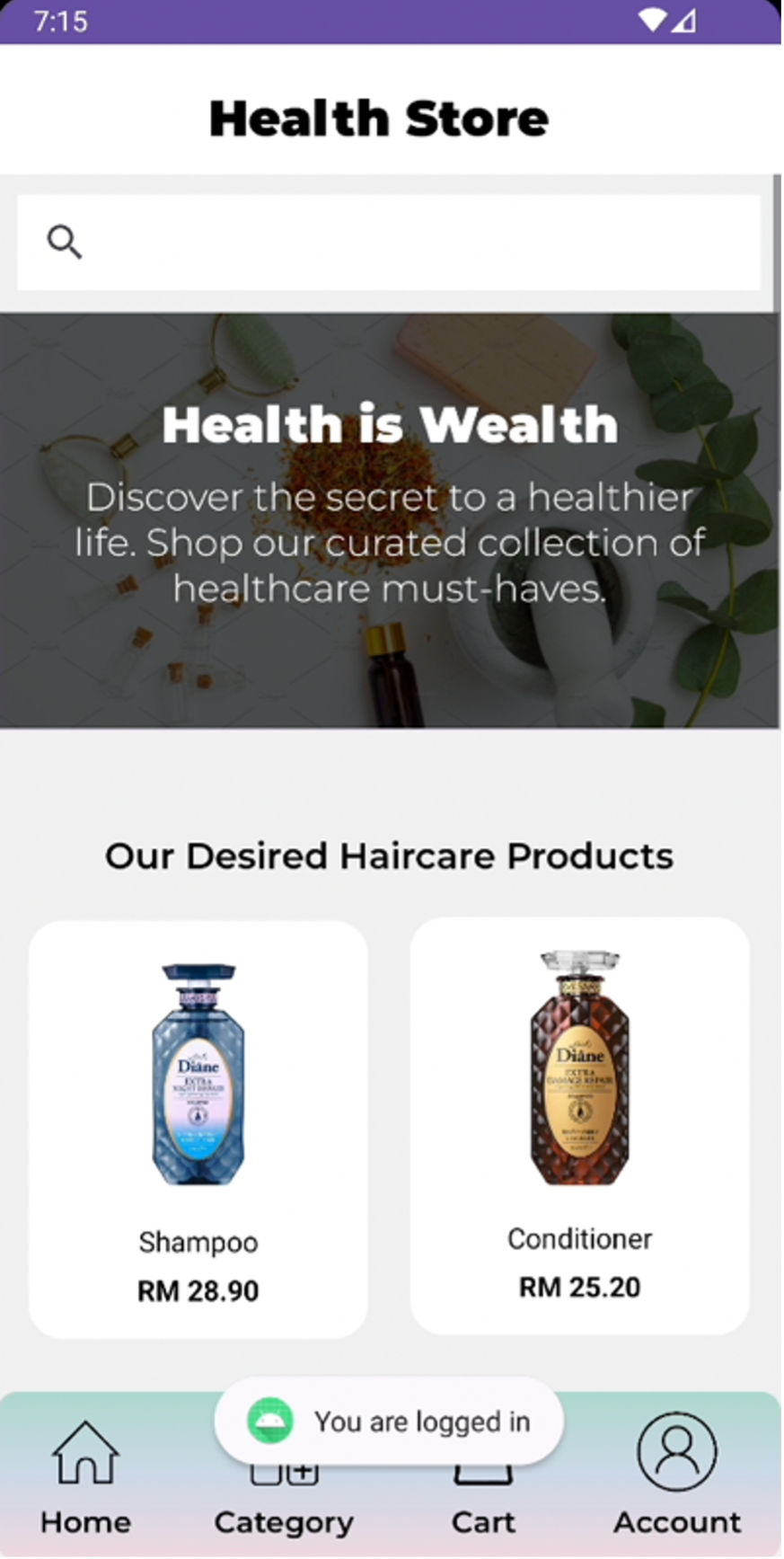
1.2 Handling Incorrect User Information when Login
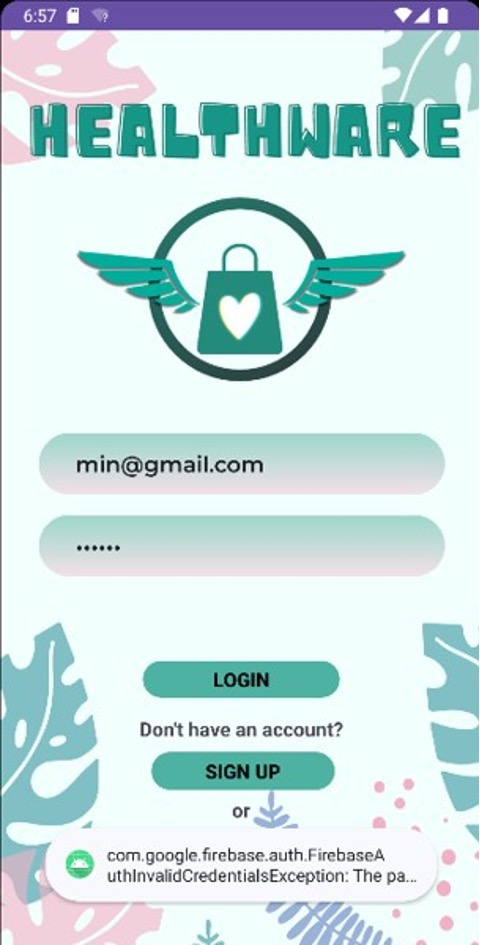
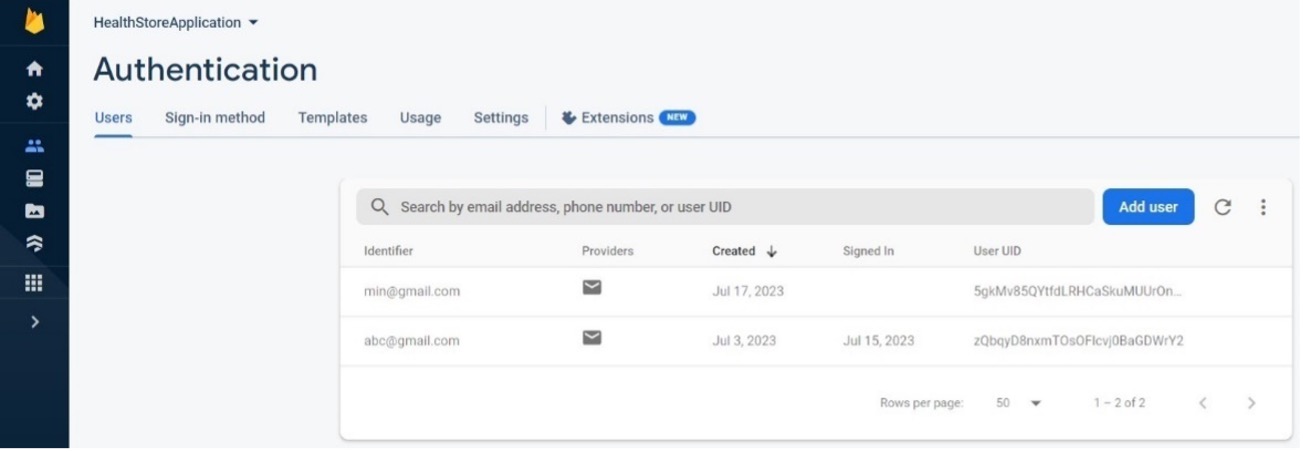
If the credentials are wrong, a toast message pops up saying, “There is no user record for this identifier. The user may have been deleted.” This happens because we’re using
, a cloud-based NoSQL database that syncs and stores data in real time. It ensures your data is always up-to-date during login (Figure 1.5) and improves authentication reliability.2. Home Page
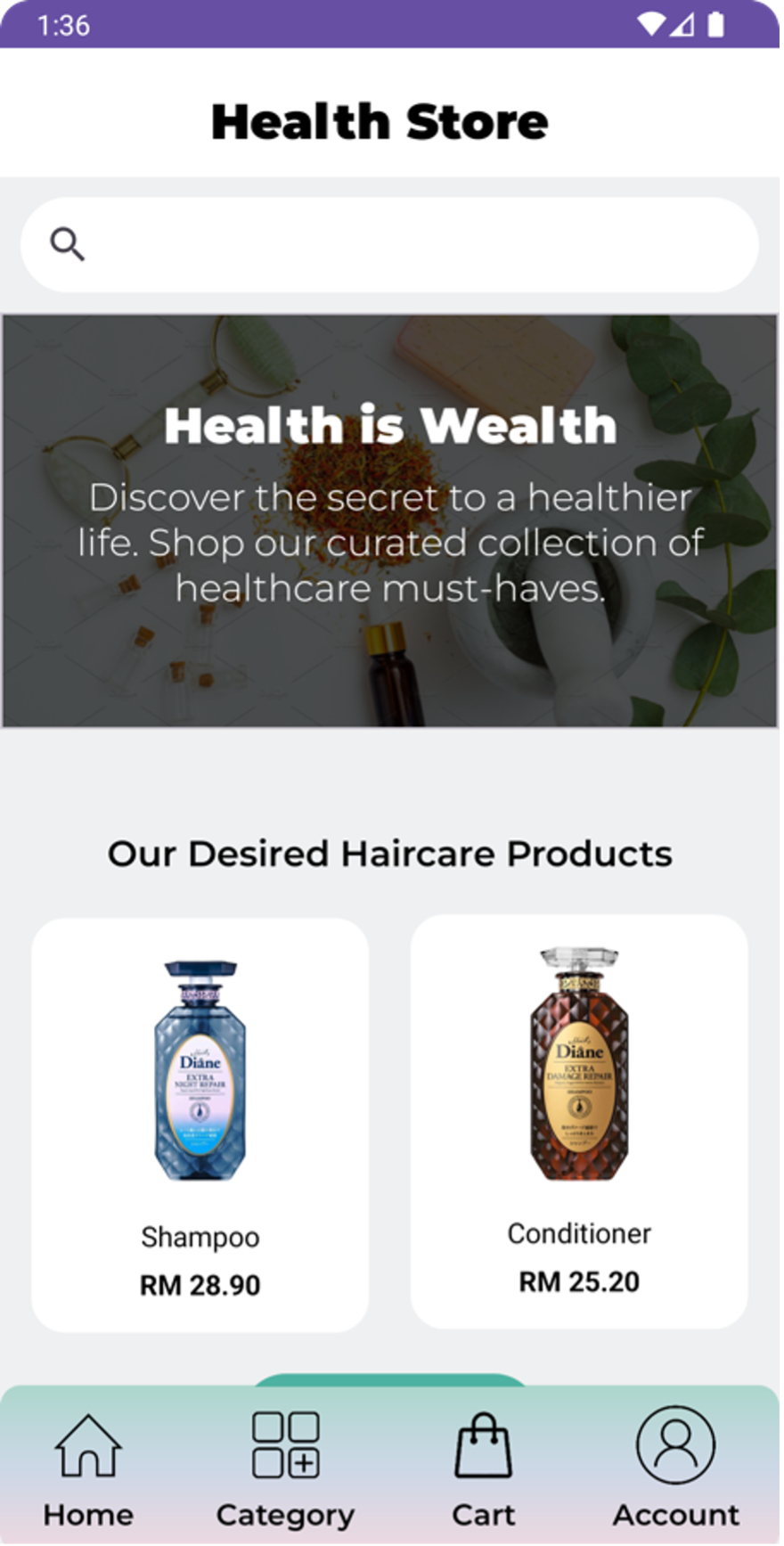
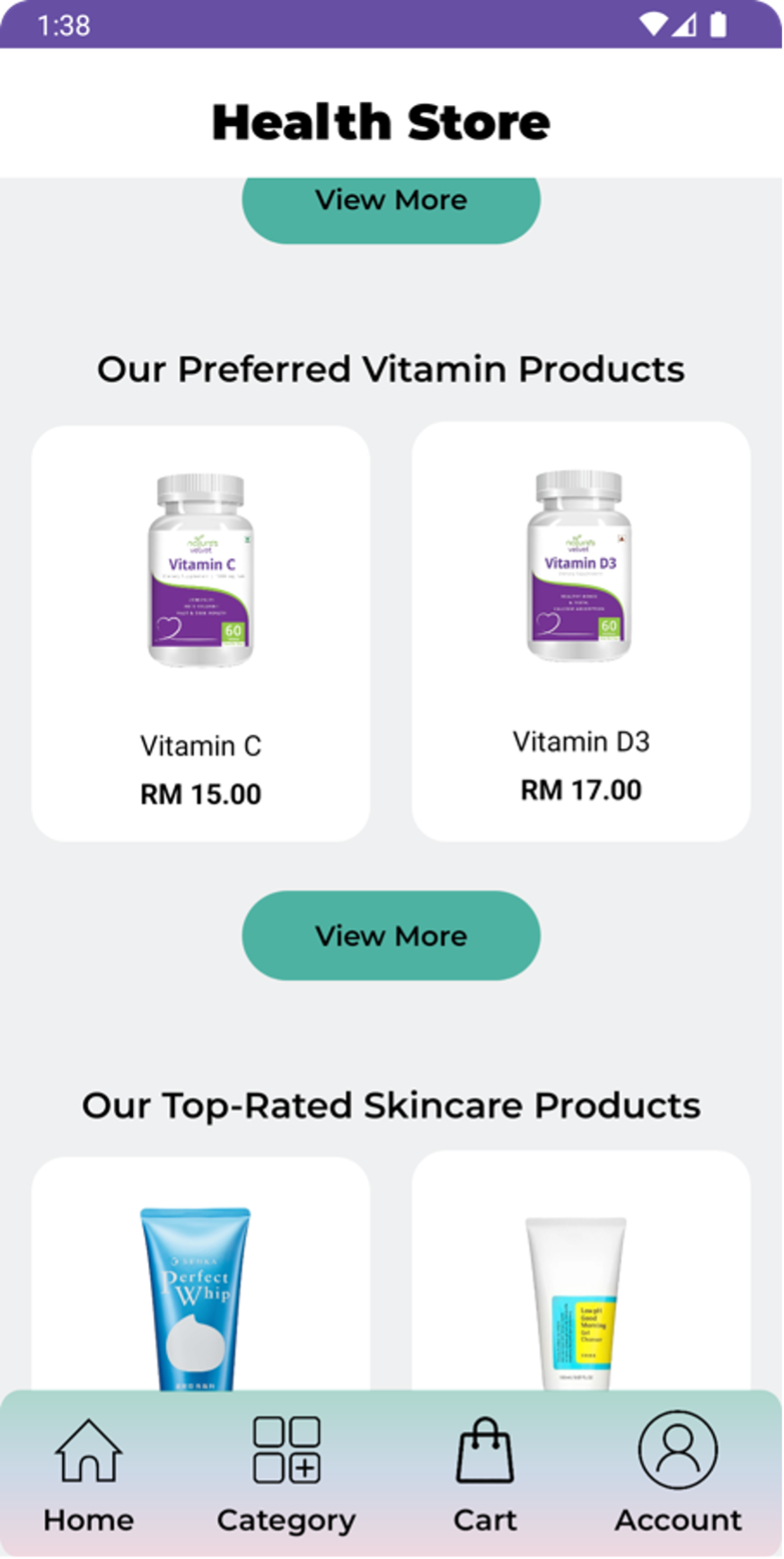
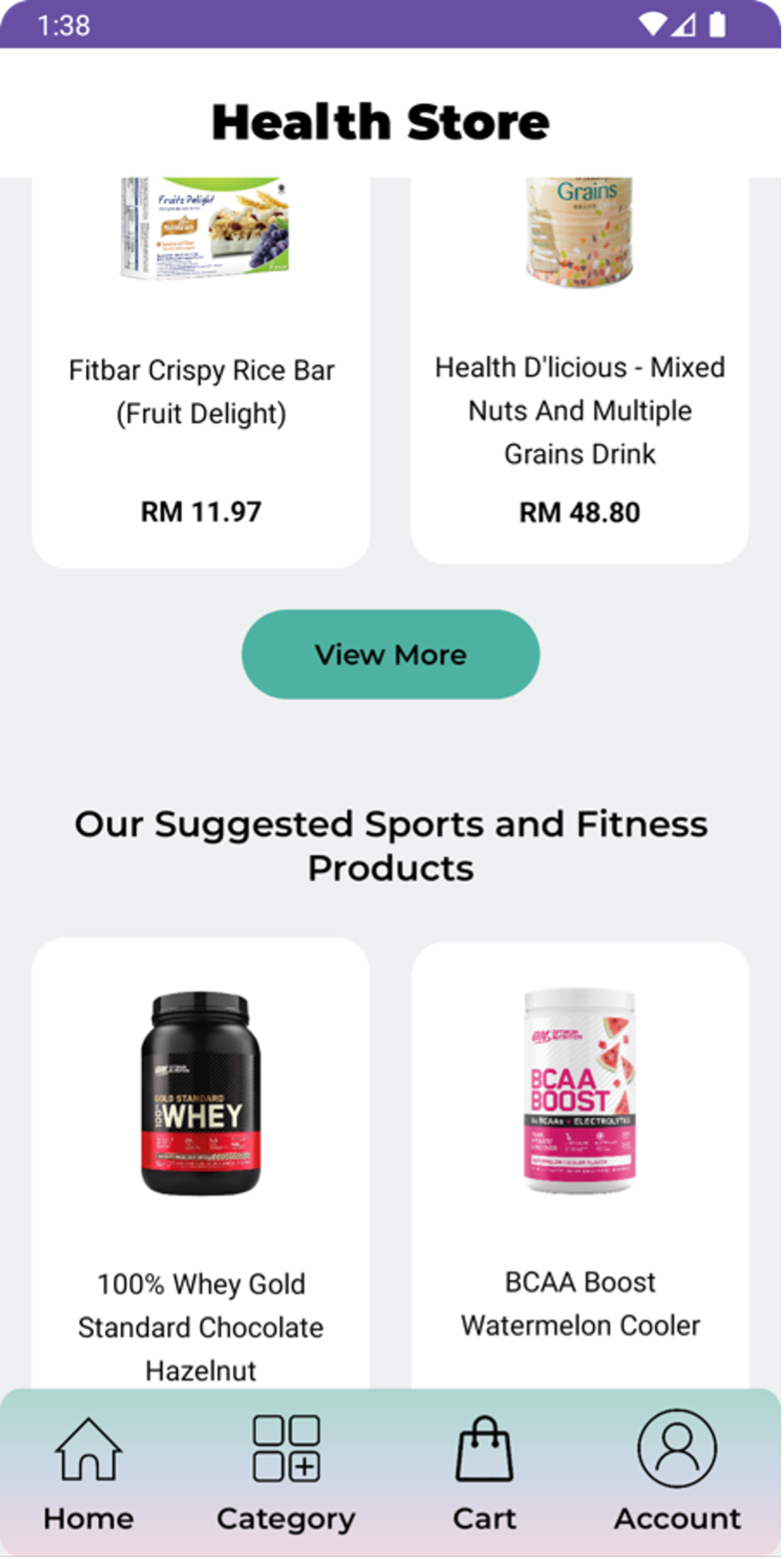
3. Category Page
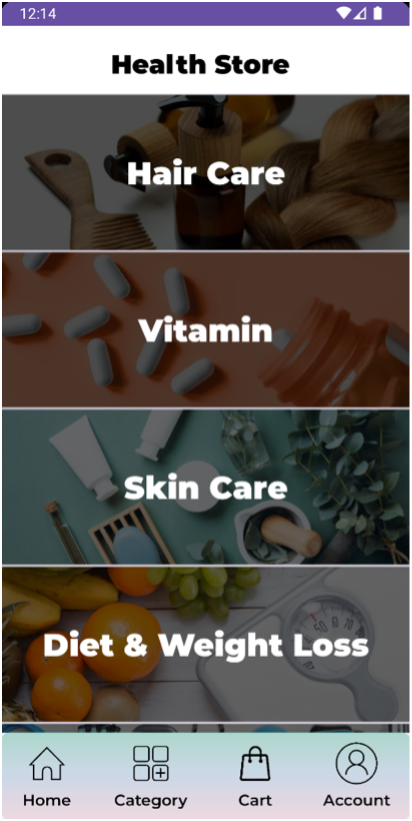
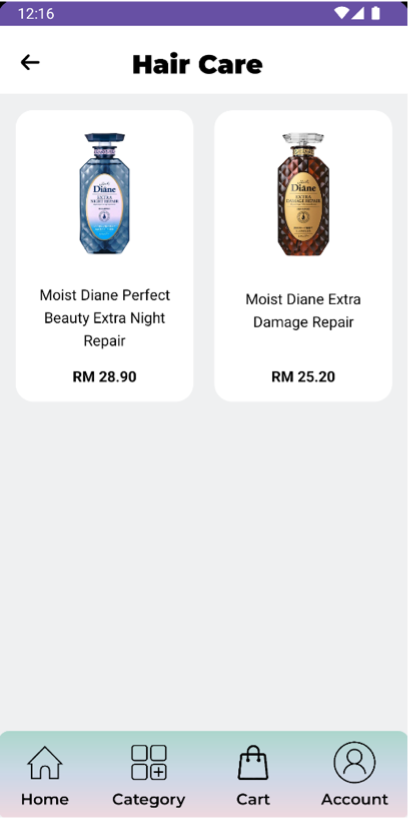
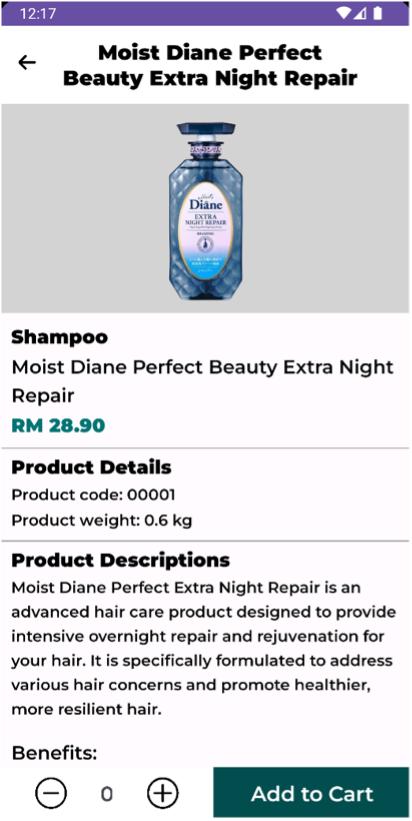
4. Cart Page
4.1 Add items into cart
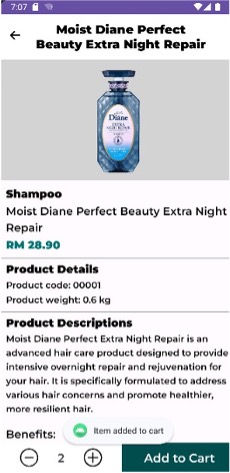
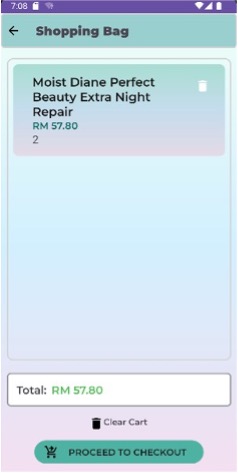
4.2 Remove items from cart
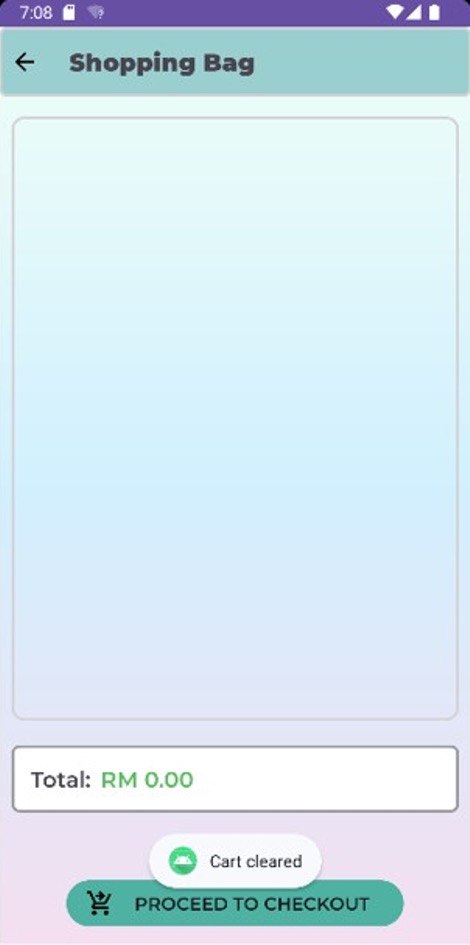
4.3 Checkout and Payment
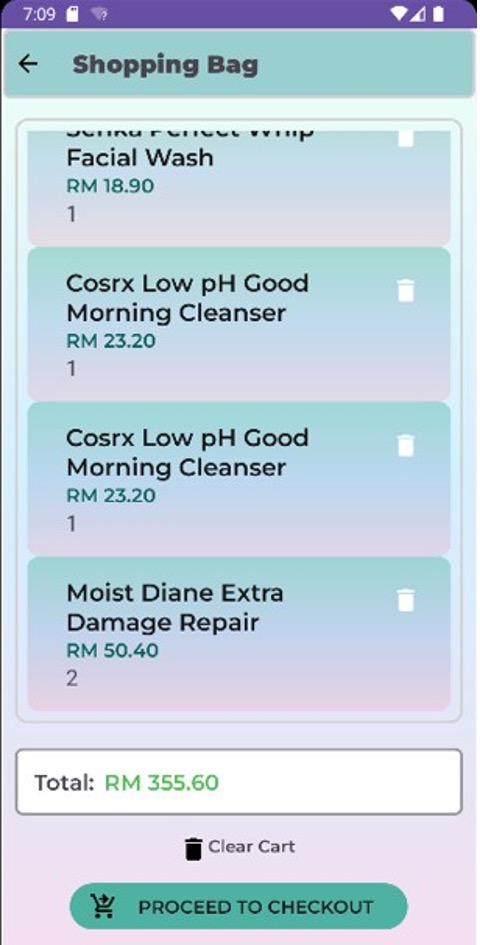
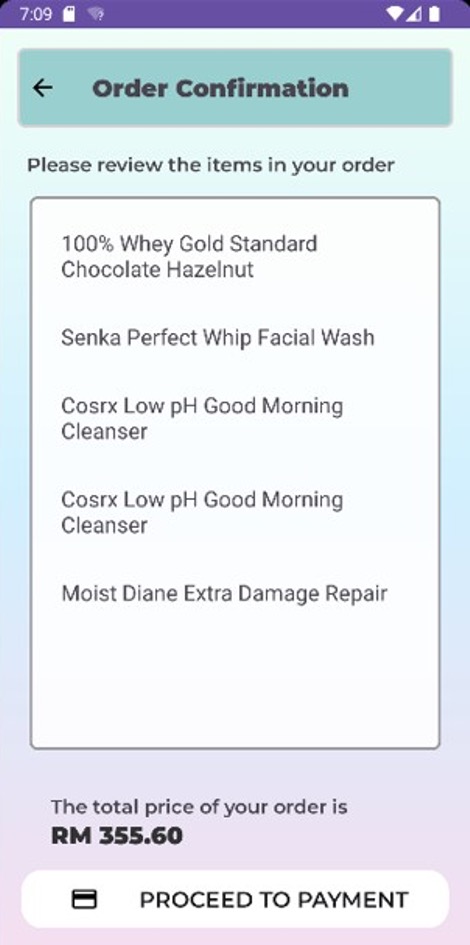
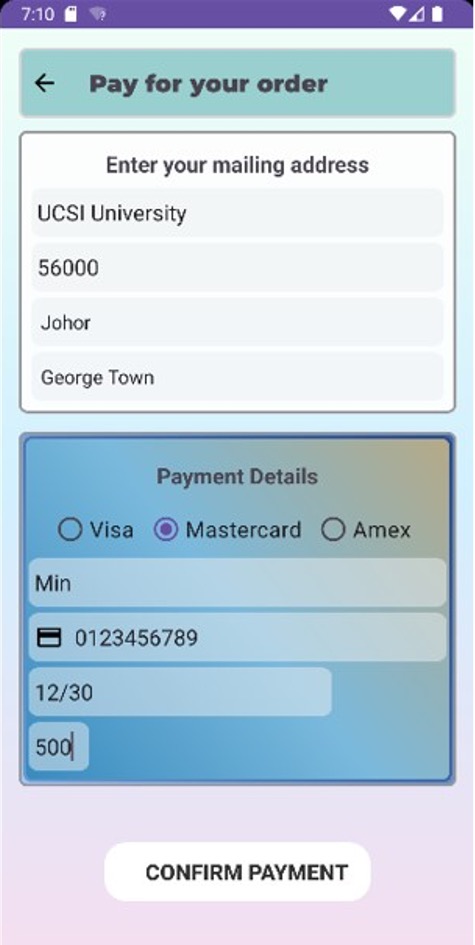
public void updateTotalAmount() {
double totalAmount = calculateTotalAmount();
DecimalFormat decimalFormat = new DecimalFormat("0.00");
String formattedTotalAmount = decimalFormat.format(totalAmount);
textViewTotalAmount.setText("RM " + formattedTotalAmount);
}
private double calculateTotalAmount() {
double totalAmount = 0;
for (CartItem cartItem : cartItems) {
totalAmount += cartItem.getItemPrice() * cartItem.getQuantity();
}
DecimalFormat decimalFormat = new DecimalFormat("0.00");
String formattedTotalAmount = decimalFormat.format(totalAmount);
textViewTotalAmount.setText("RM " + formattedTotalAmount);
return totalAmount;
}
The
method clears the cart, grabs the user ID, locates the user via Firebase, and updates the total. A toast confirms the action. The method adds up the cart total by multiplying itemPrice and quantity, then shows it on the screen. The method does the same but returns the total as a raw double.private void addToOrder(String selectedState, String selectedCity) {
if (mAuth.getCurrentUser() != null) {
String userId = mAuth.getCurrentUser().getUid();
String currentDate = DateFormat.getDateTimeInstance().format(new Date());
String orderId = ordersRef.push().getKey();
Map<String, Object> orderDetails = new HashMap<>();
orderDetails.put("state", selectedState);
orderDetails.put("city", selectedCity);
orderDetails.put("orderDate", currentDate);
orderDetails.put("orderId", orderId);
double totalPrice = 0;
int totalQuantity = 0;
cartRef.addListenerForSingleValueEvent(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot snapshot) {
if (snapshot.exists()) {
for (DataSnapshot cartItemSnapshot : snapshot.getChildren()) {
CartItem cartItem = cartItemSnapshot.getValue(CartItem.class);
String cartItemId = cartItemSnapshot.getKey();
// Add the cart item to the order details
ordersRef.child(orderId).child("items").child(cartItemId).setValue(cartItem);
double itemPrice = cartItem.getItemPrice();
int quantity = cartItem.getQuantity();
double itemTotal = itemPrice * quantity;
totalPrice += itemTotal; // Update the total price
}
ordersRef.child(orderId).child("totalPrice").setValue(totalPrice);
cartRef.removeValue(); // Clear cart after successful order
}
}
@Override
public void onCancelled(@NonNull DatabaseError error) {
Toast.makeText(Payment.this, "Failed to retrieve cart items", Toast.LENGTH_SHORT).show();
}
});
ordersRef.child(orderId).setValue(orderDetails); // Save order details in Firebase
}
// Redirect to the order confirmation screen
Intent intent = new Intent(Payment.this, OrderConfirmation.class);
startActivity(intent);
// Handle the progress dialog and confirmation message
int progressDelayMillis = 5000; // Closing progress dialog after 5 seconds
new CountDownTimer(progressDelayMillis, progressDelayMillis) {
@Override
public void onTick(long millisUntilFinished) {}
@Override
public void onFinish() {
progressDialog.dismiss();
}
}.start();
int toastDelayMillis = 1000; // Delay before showing confirmation toast
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
Toast.makeText(getApplicationContext(), "Your order has been confirmed!", Toast.LENGTH_LONG).show();
}
}, toastDelayMillis);
}
The
method saves the order details to Firebase after payment. It grabs the cart items, calculates the total, and stores everything (like state, city, order date) in Firebase under the user’s orders. Then, it clears the cart and redirects the user to the OrderConfirmation screen after a quick progress update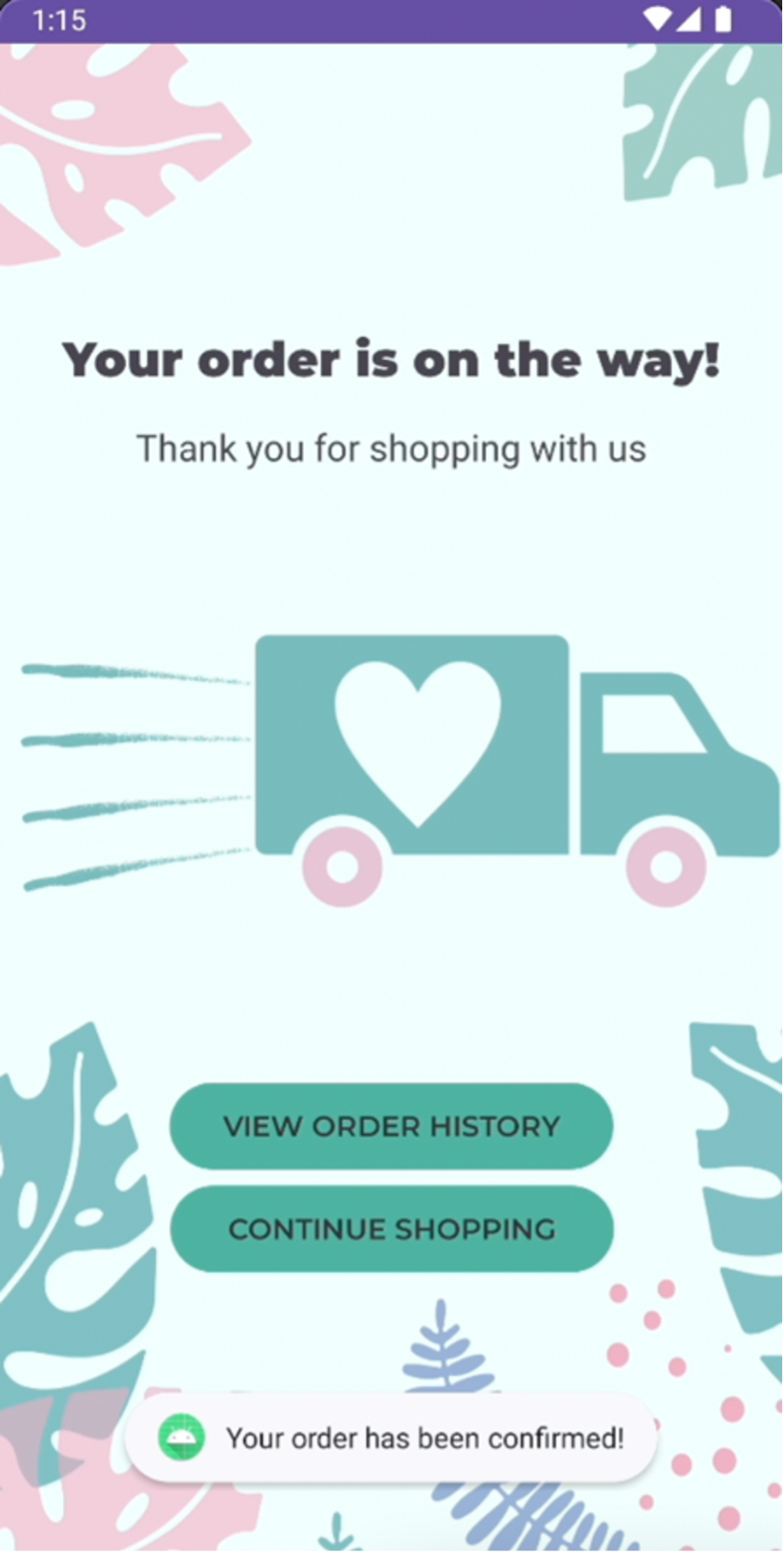
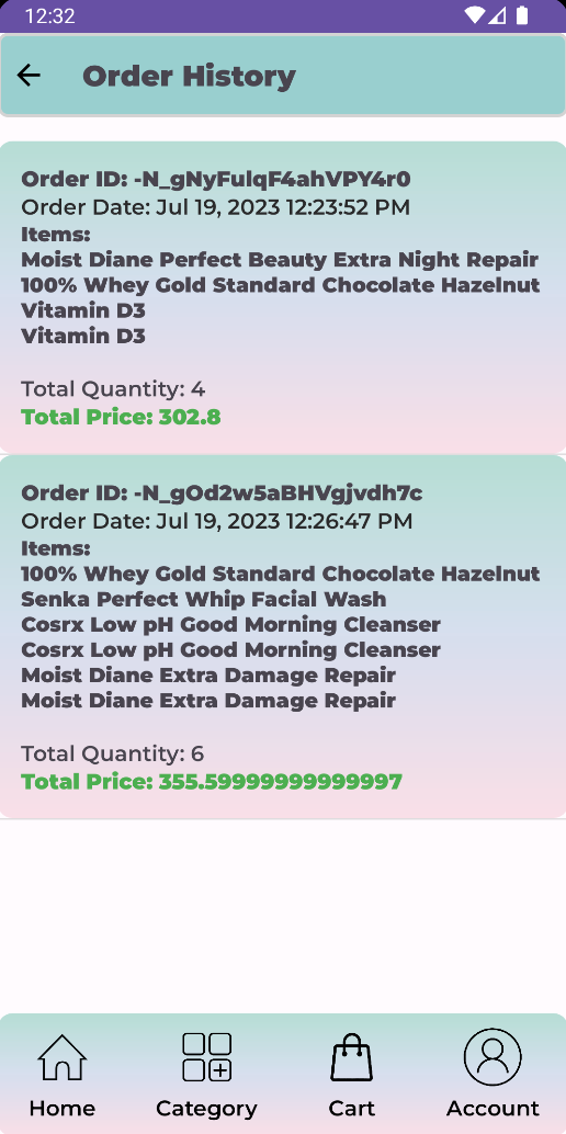
private void fetchOrderHistory(String userId) {
ordersRef.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(DataSnapshot dataSnapshot) {
List<Order> orderList = new ArrayList<>();
for (DataSnapshot orderSnapshot : dataSnapshot.getChildren()) {
String orderId = orderSnapshot.child("orderId").getValue(String.class);
String orderDate = orderSnapshot.child("orderDate").getValue(String.class);
DataSnapshot itemsSnapshot = orderSnapshot.child("items");
List<String> orderItems = new ArrayList<>();
double totalOrderPrice = 0;
int totalQuantity = 0;
for (DataSnapshot itemSnapshot : itemsSnapshot.getChildren()) {
String itemName = itemSnapshot.child("itemName").getValue(String.class);
int quantity = itemSnapshot.child("quantity").getValue(Integer.class);
double itemPrice = itemSnapshot.child("itemPrice").getValue(Double.class);
double itemTotalPrice = itemPrice * quantity;
totalOrderPrice += itemTotalPrice;
totalQuantity += quantity;
orderItems.add(itemName);
}
Order order = new Order(orderId, orderItems, totalOrderPrice, totalQuantity, orderDate);
orderList.add(order);
}
OrderAdapter adapter = new OrderAdapter(OrderHistory.this, orderList);
listViewOrders.setAdapter(adapter);
}
The
method is used to fetch the order history from Firebase Realtime Database and it iterates over order snapshots and retrieves order ID, order date, and items for each order. “Order” object is also created and added to “orderList” and “OrderAdapter” is set up with “orderList” and assigns it to “listViewOrders” to display the order history.5. Account Page
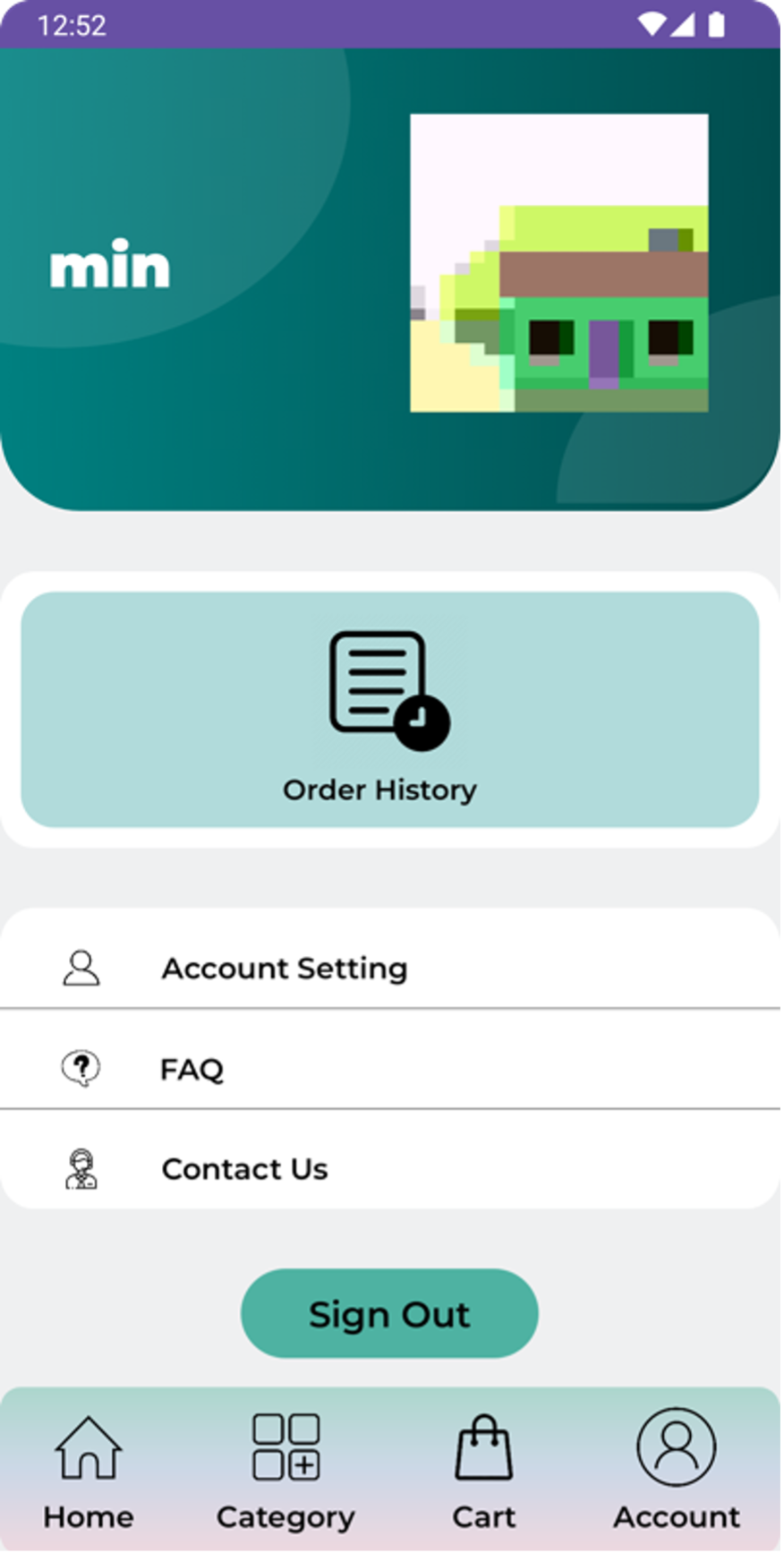
5.1 Update profile picture
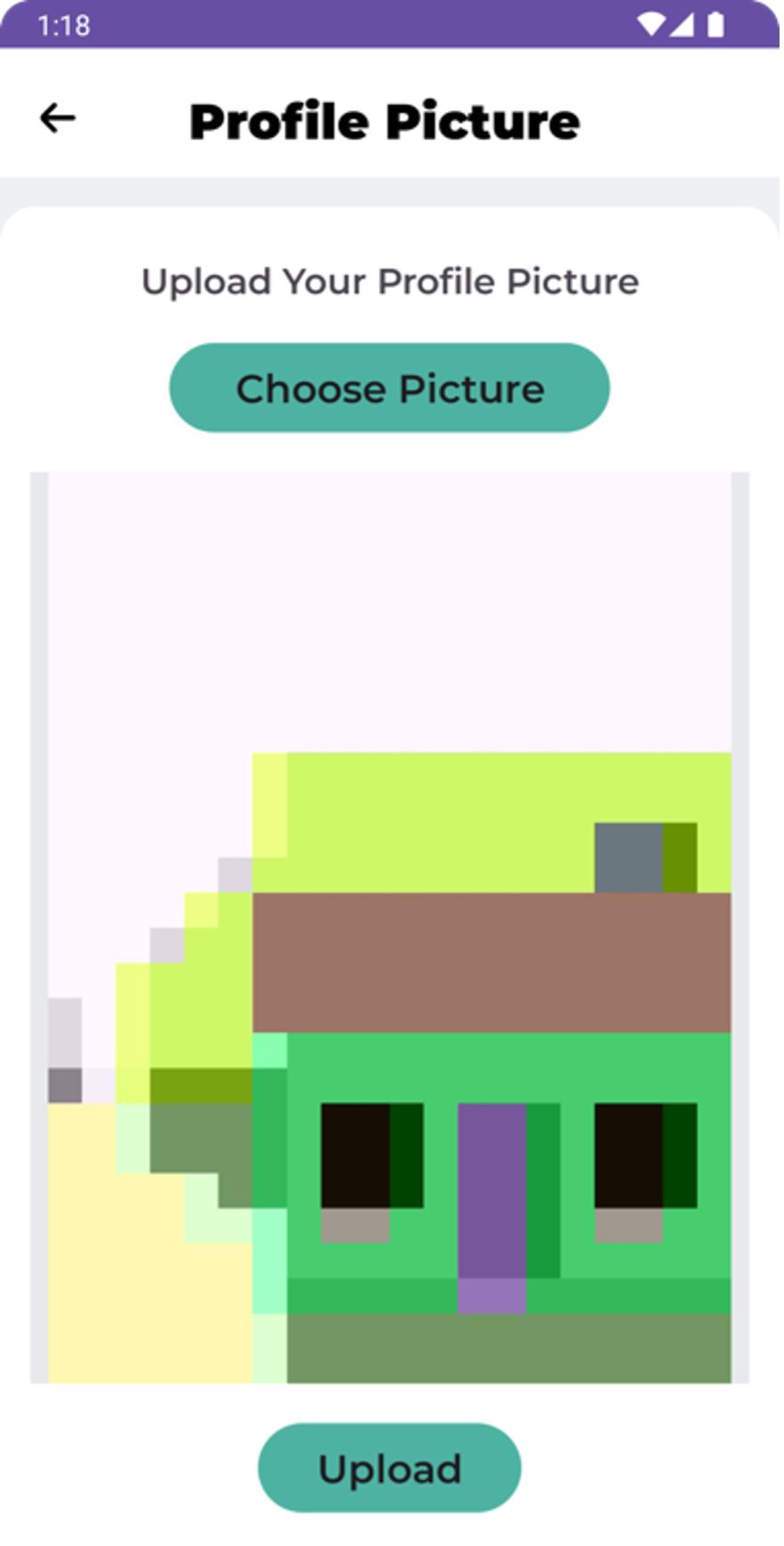
5.2 Update profile information
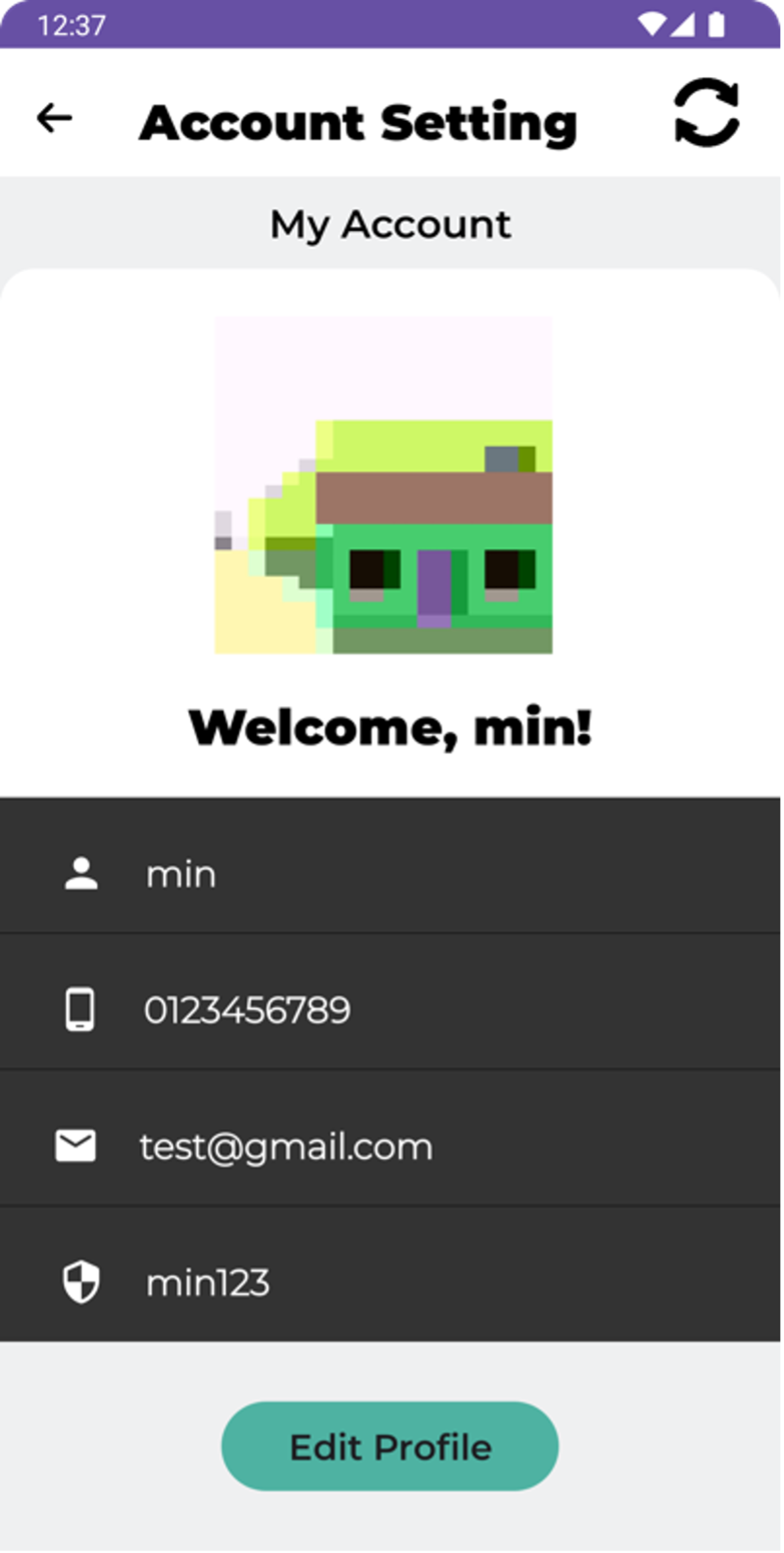
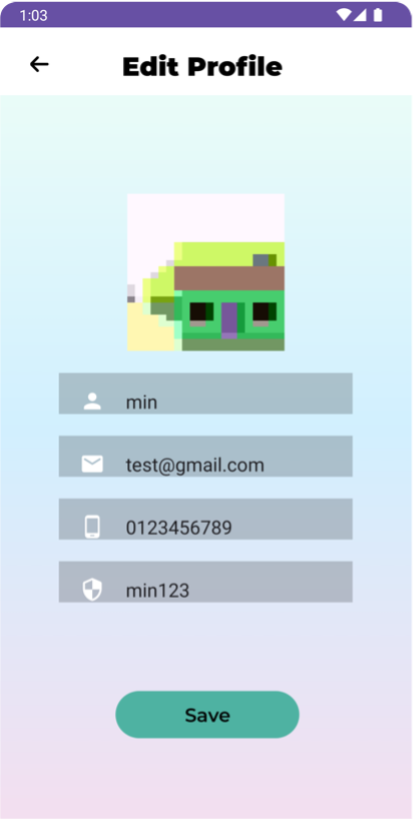
5.3 Logout
Click the “Sign Out” button to log out of the app. A toast message pops up saying, “You are signed out!“
7. Guest Mode
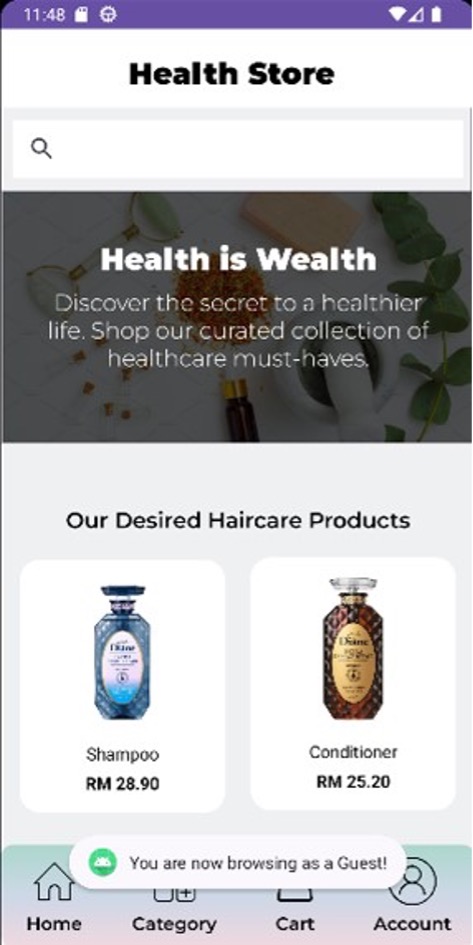
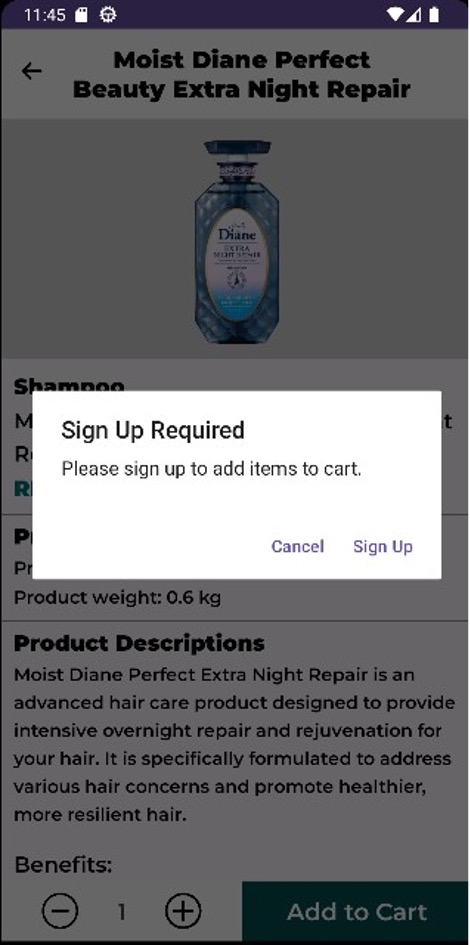
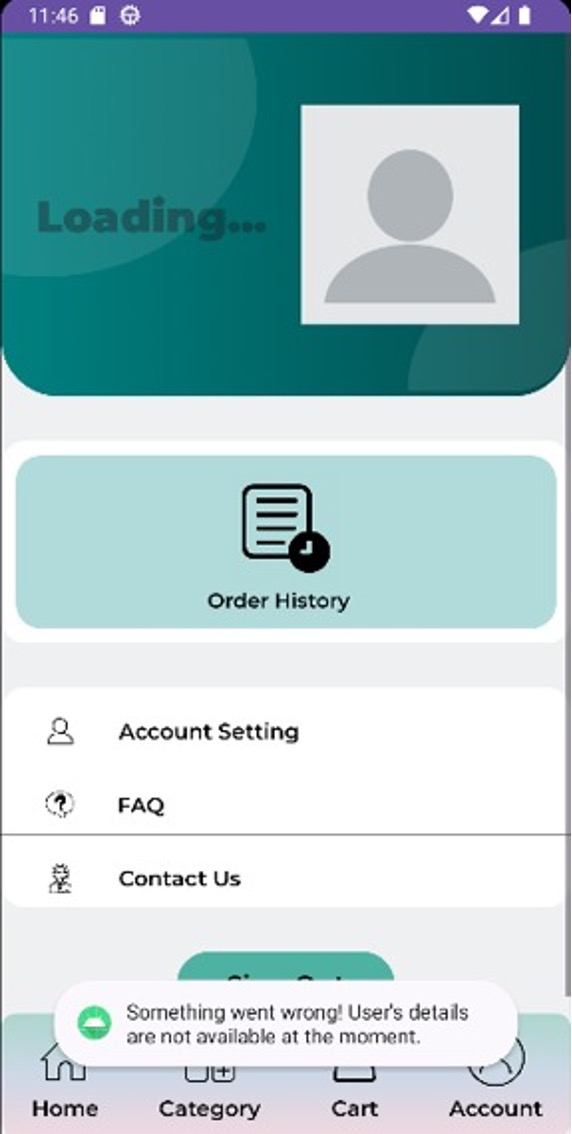
Conclusion
The Healthware app consists of 37 Java classes, which together form the foundation for a fully functional healthcare ordering system. All the project requirements have been successfully met and implemented. This project marked my first experience in creating an e-commerce shopping system from the ground up using Java, Android Studio, and Firebase. It was a great learning journey, and I’m really proud of the final result.
Tech Stack
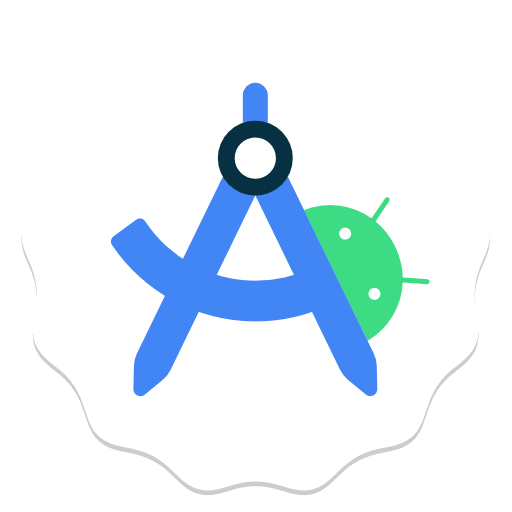
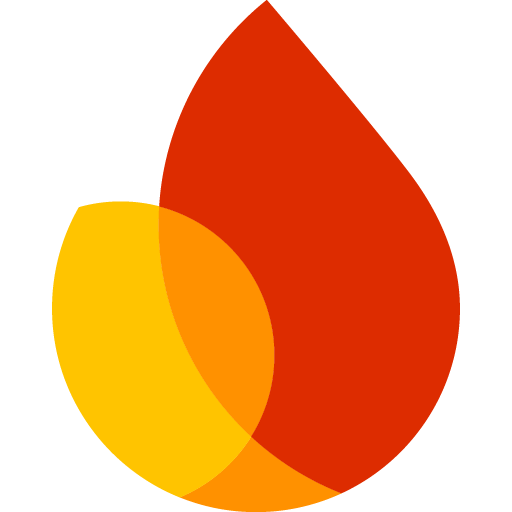
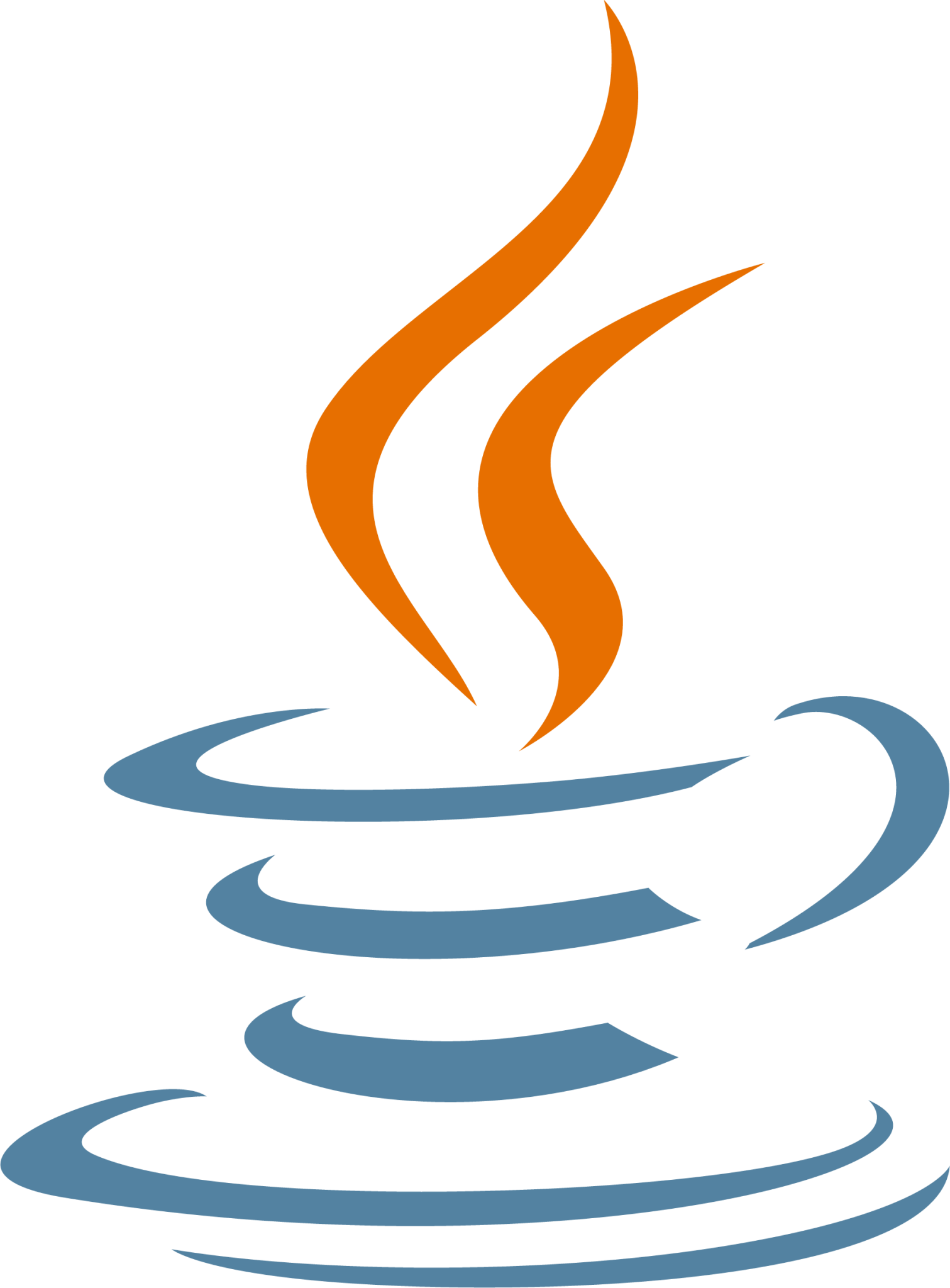