Important note: Feel free to admire my final year project, but please resist the urge to “borrow” it. I’ve spent countless sleepless nights perfecting this masterpiece. So unless you’re planning to give me full credit (and maybe a yacht), kindly keep your hands off.
Introduction
We all know picking the perfect fruit can be a hit-or-miss game, relying on how shiny, squishy, or “perfectly” round it looks. But let’s face it, this method is slow and often misleading. Enter AI! With the rise of machine learning, we now have an Android app that takes the guesswork out of fruit shopping. It analyzes key traits—color, shape, size, defects—to instantly tell you if that apple’s ripe or ready to toss. This app doesn’t just save time; it helps reduce food waste, promotes healthier choices, and educates us on what truly makes fruit fresh. This page dives into how current apps stack up, what’s missing, and how we can make fruit selection smarter than ever.
Objectives
- Analyze existing apps to spot what works and what doesn’t in fruit quality detection.
- Design a super user-friendly Android app for easy fruit freshness checks.
- Build the app using Android Studio, adding smarter features for better performance.
- Test the app’s usability to ensure it’s as intuitive as possible.
Methods
Design
This chapter covers the design and architecture behind FruitSense, turning the gathered requirements into a clear plan for implementation.
Flowchart
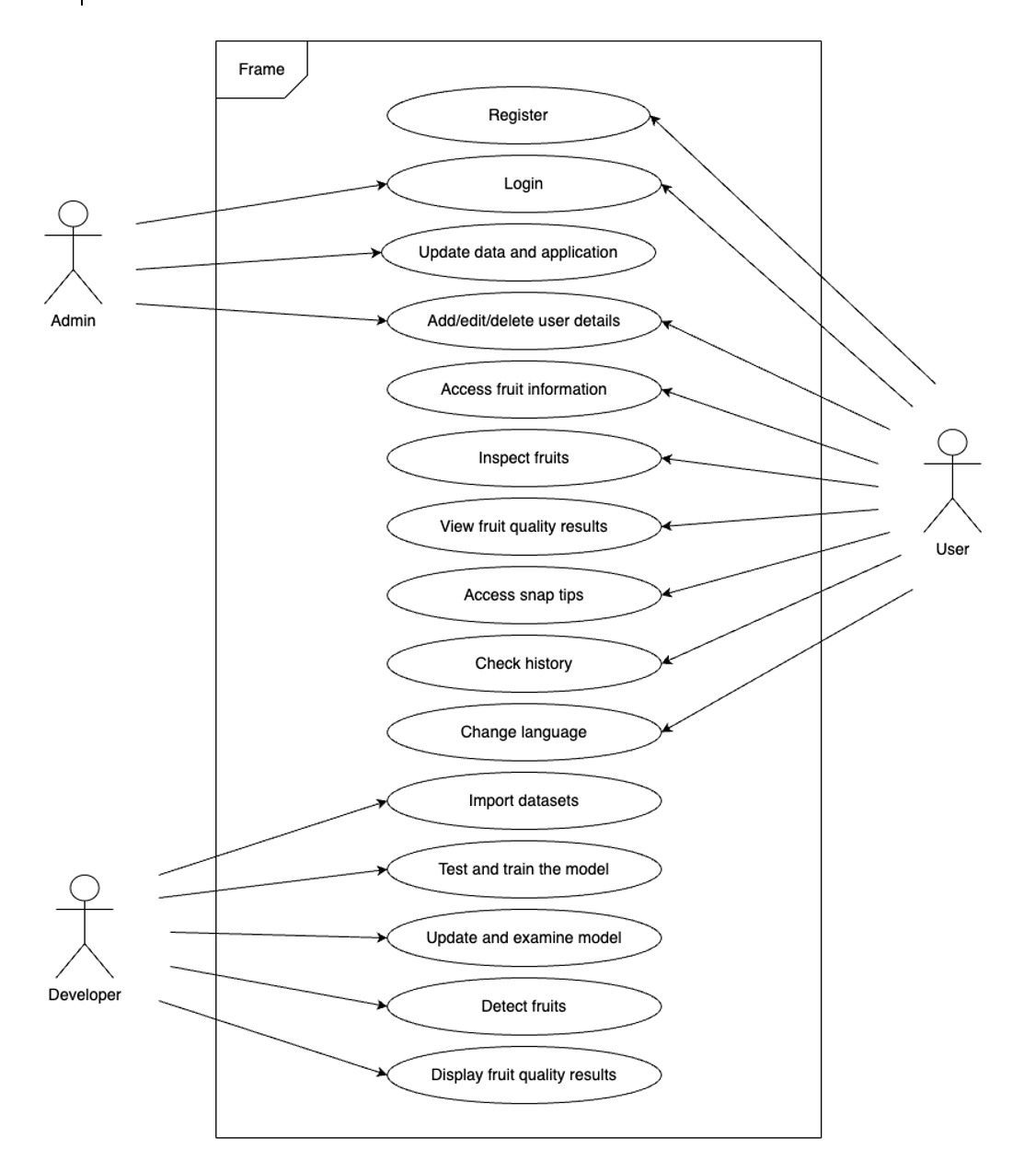
- Admins log in to manage data and user details.
- Users register, log in, and can update info, inspect fruit ripeness, check history, and change the language.
- Developers handle datasets, train the model, and display fruit quality results.
Google Teachable Machine
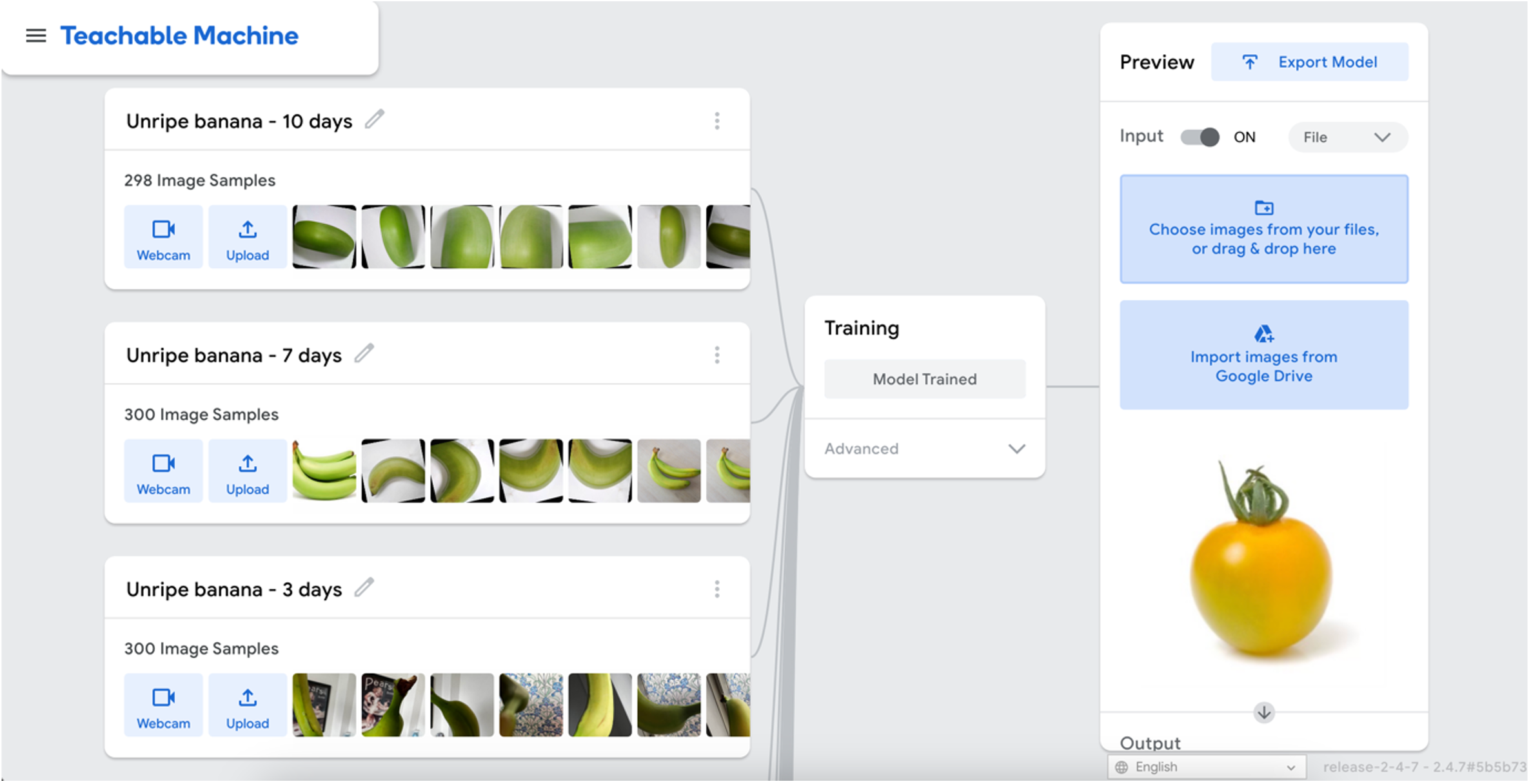
Database
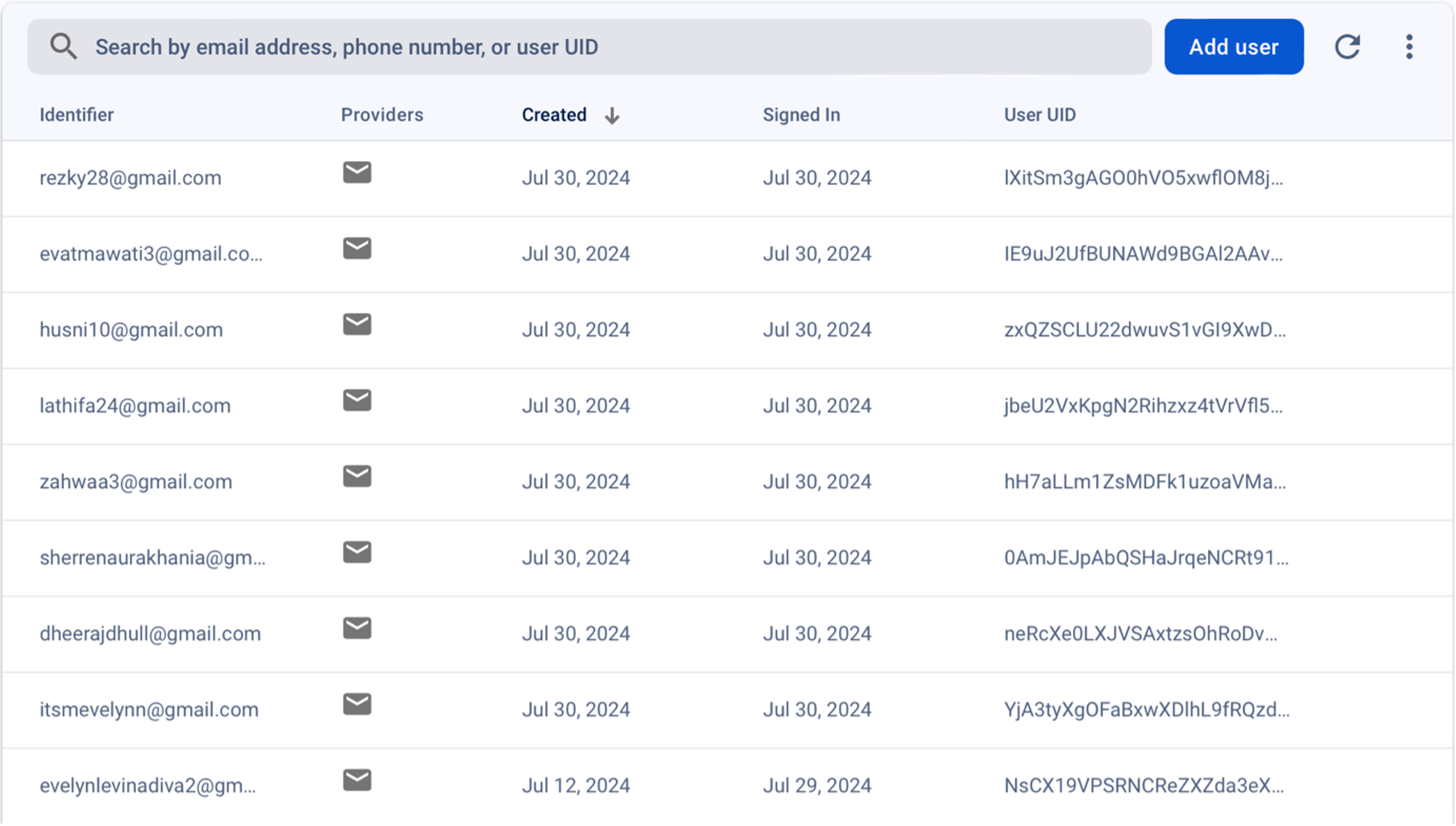
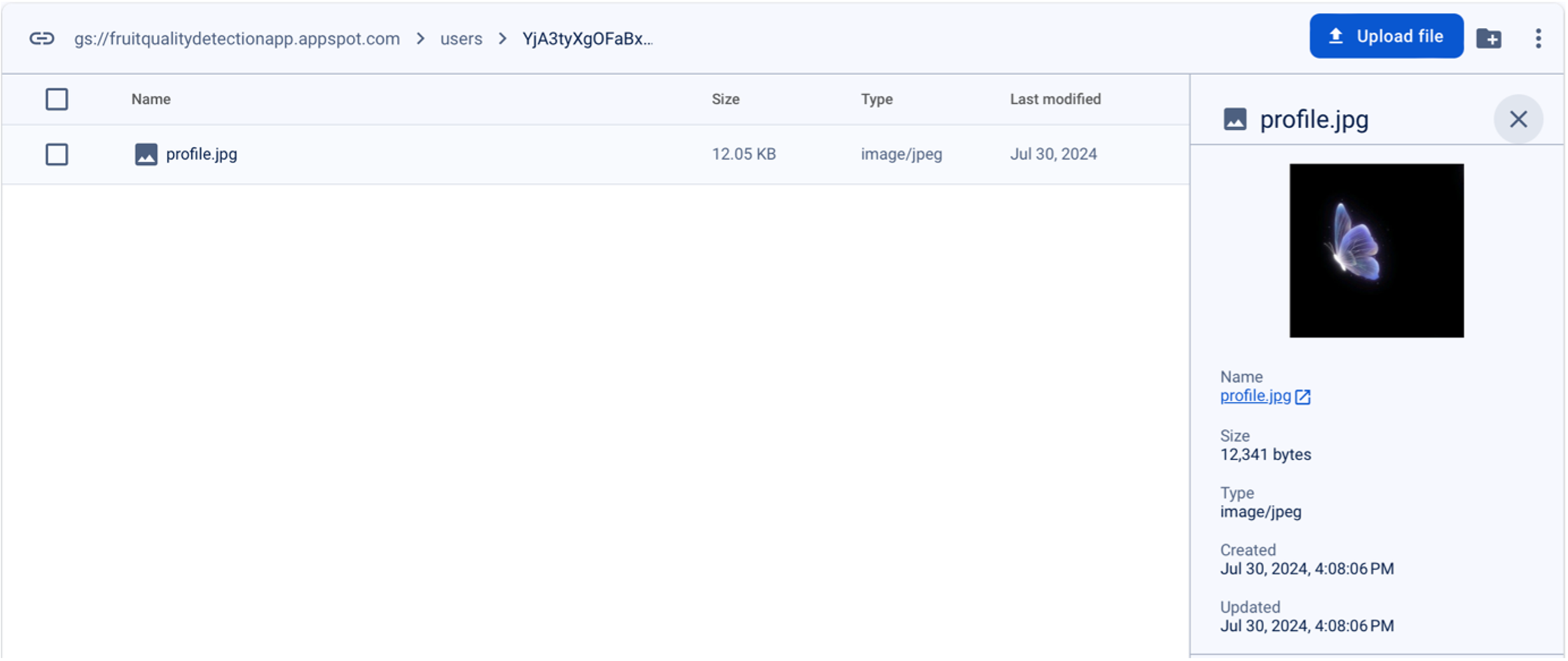
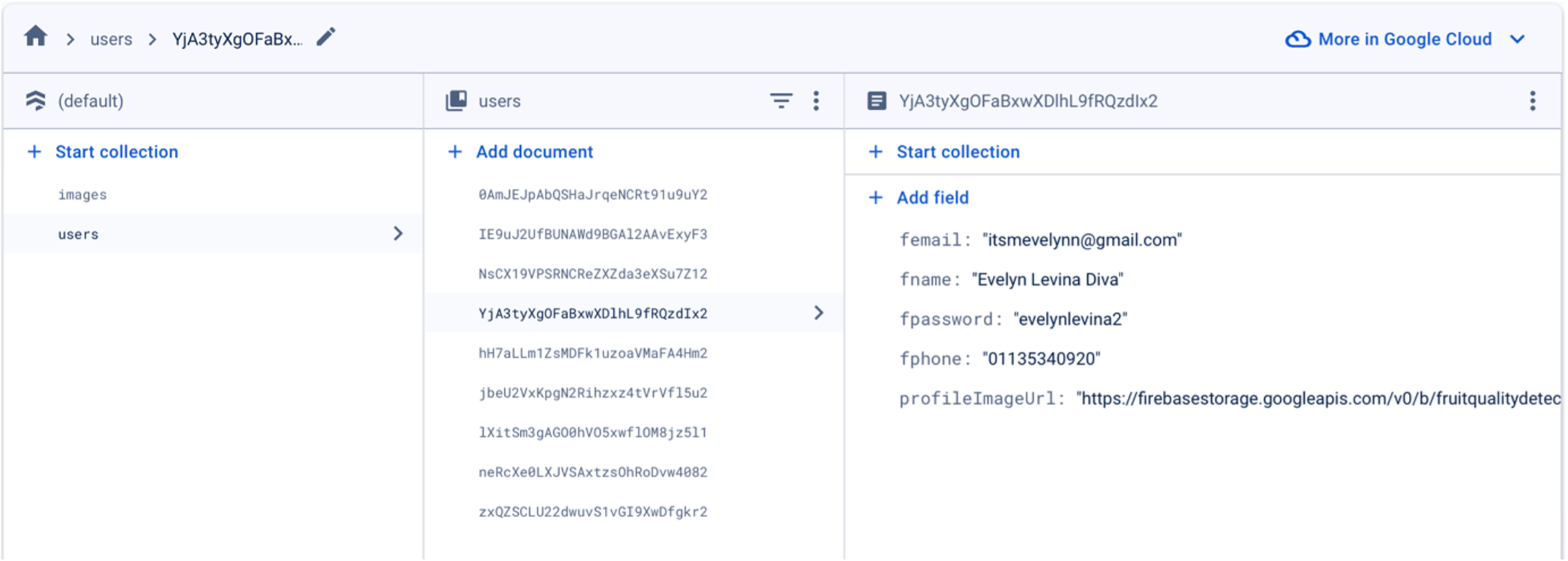
- Realtime Database: Stores all the app’s data and syncs it in real-time across devices.
- Authentication: Manages secure logins, handles password resets, and gives each user a unique ID.
- Storage: Saves profile pics and fruit images, ensuring users can upload, download, and access them easily.
- Firestore Database: Stores personal info and instantly updates changes across all devices.
mAuth = FirebaseAuth.getInstance();
mUser = mAuth.getCurrentUser();
mAuth = FirebaseAuth.getInstance();
This code initializes the Firebase Authentication instance (mAuth
) and retrieves the current authenticated user
(mUser
) to handle Firebase Authentication and user management each time the app is opened.
Print Screens and Code
1. Authentication
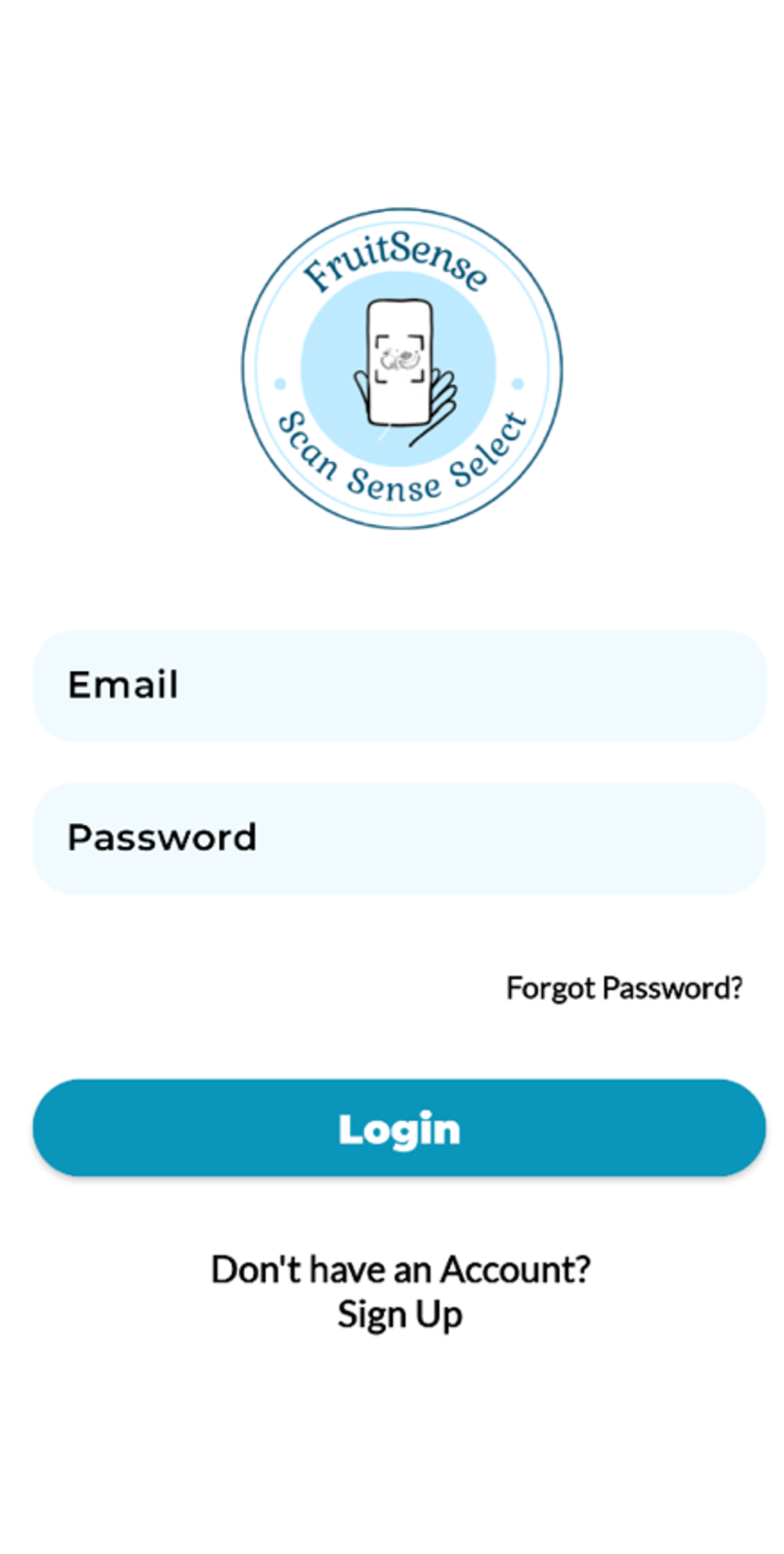
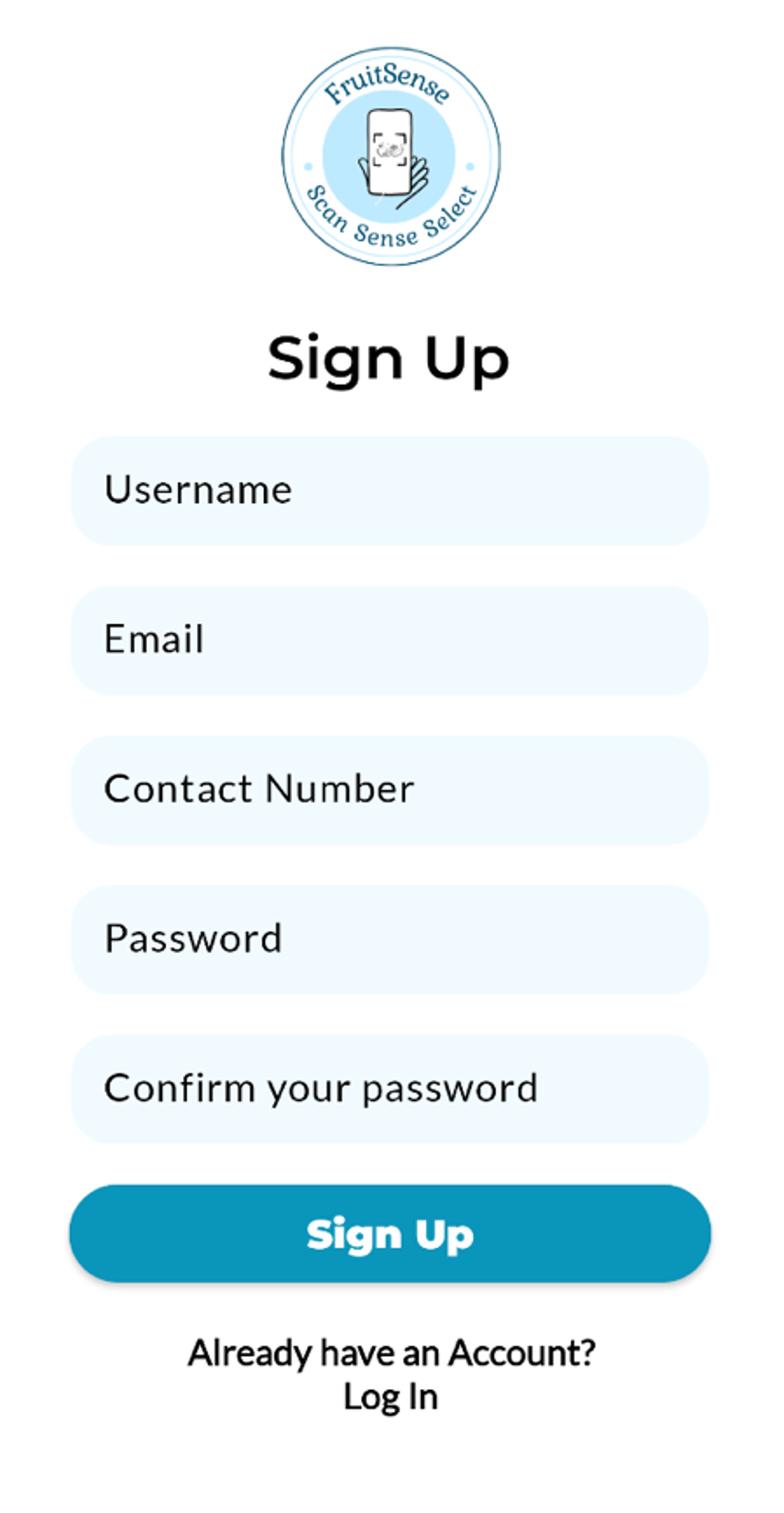
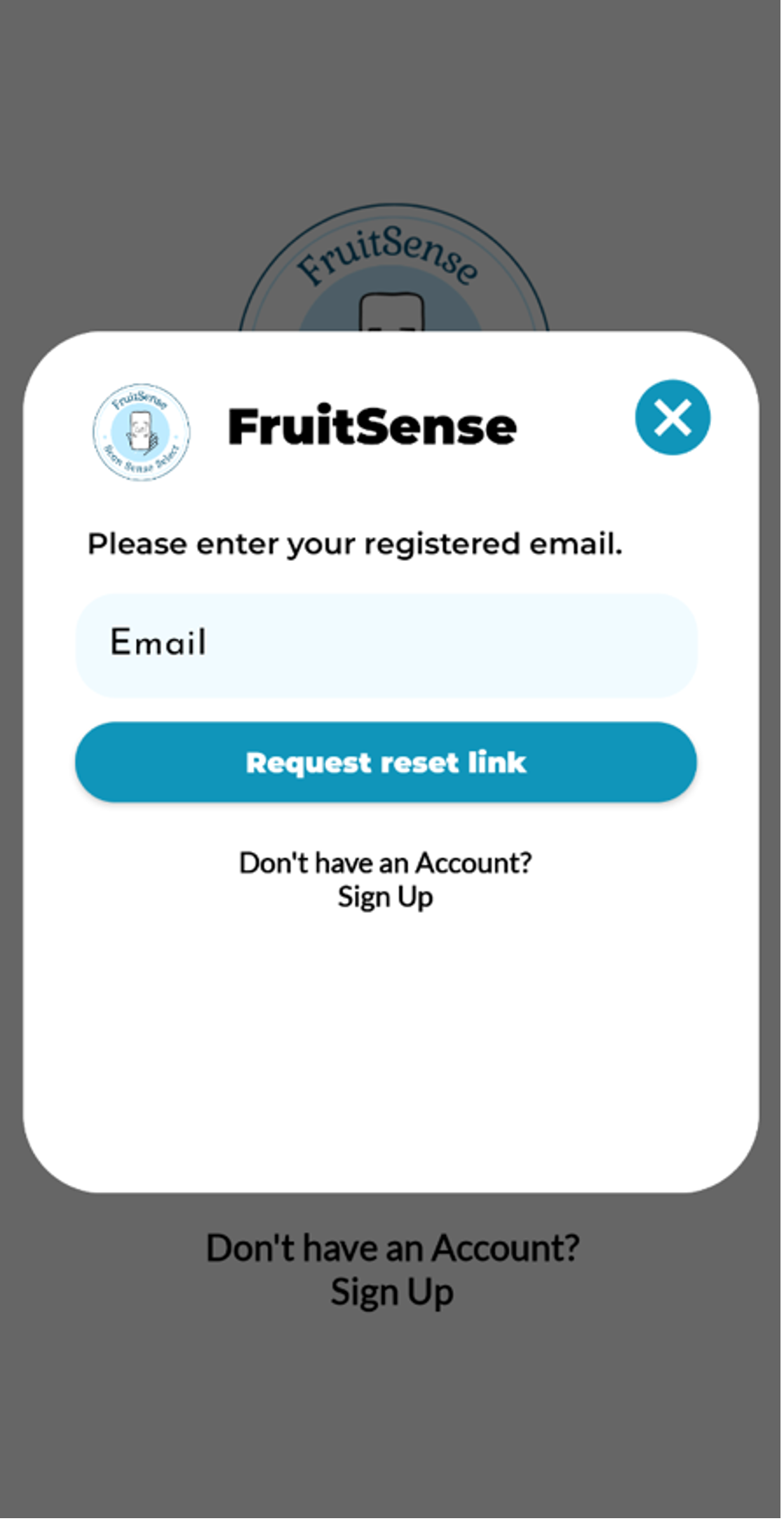
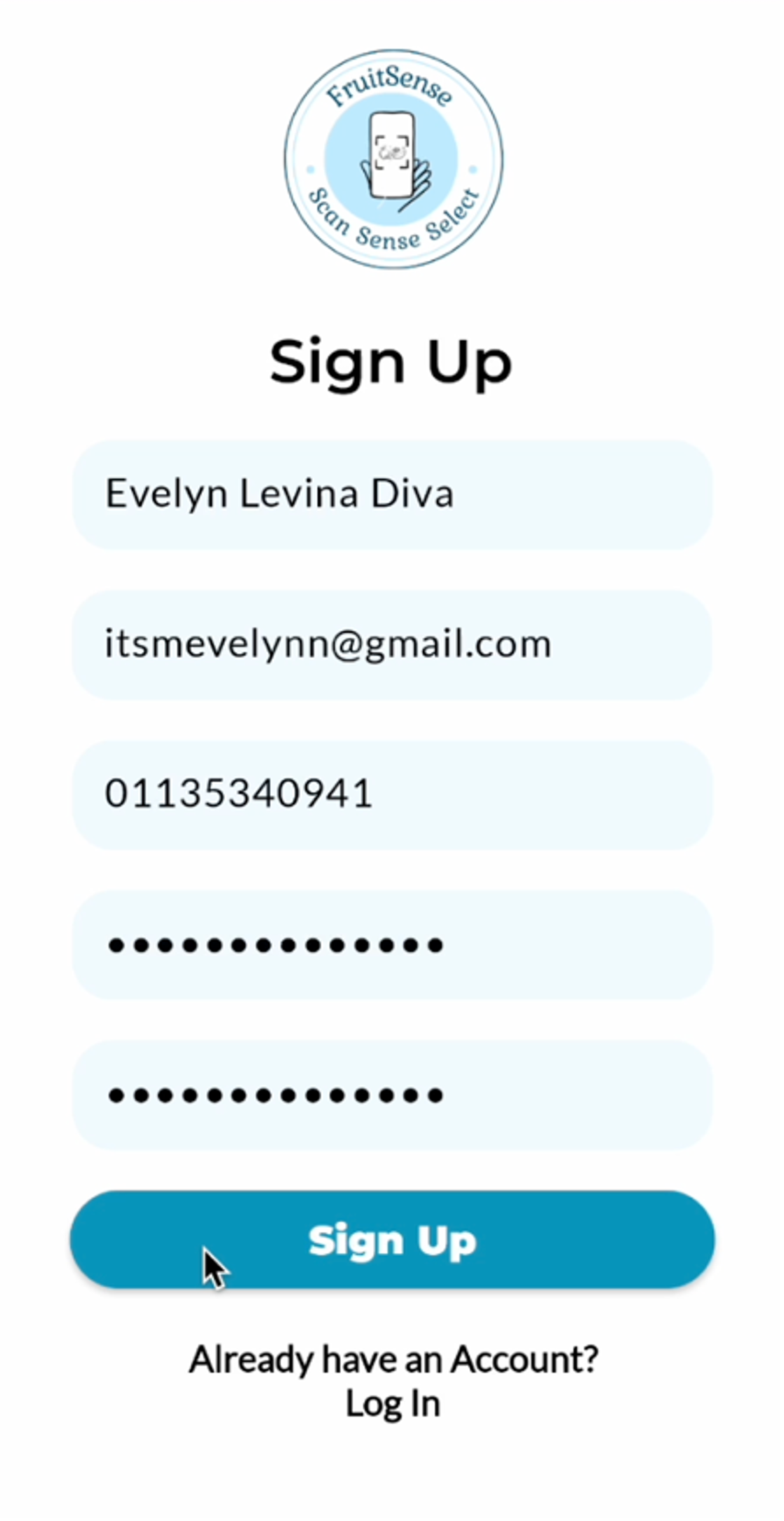
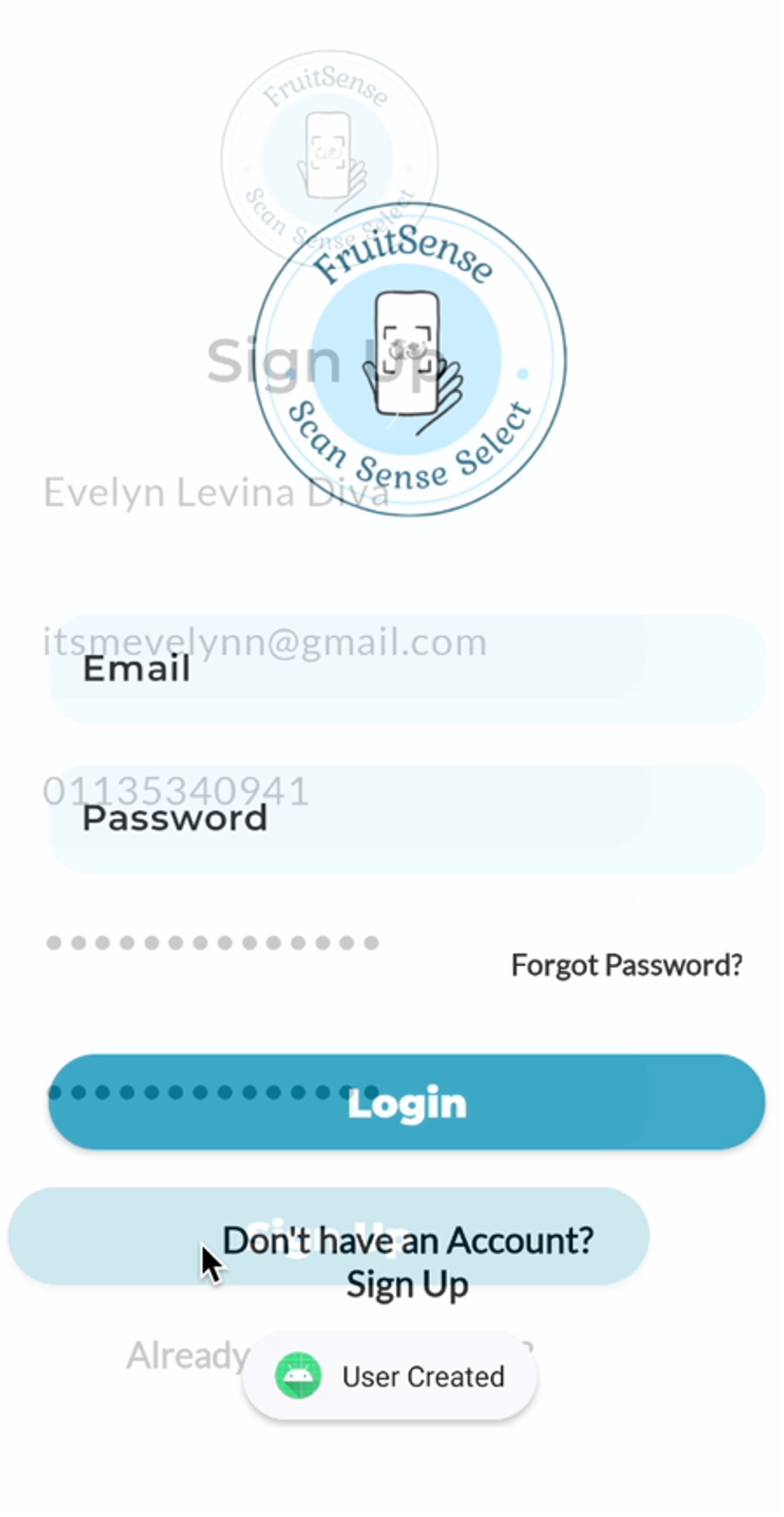
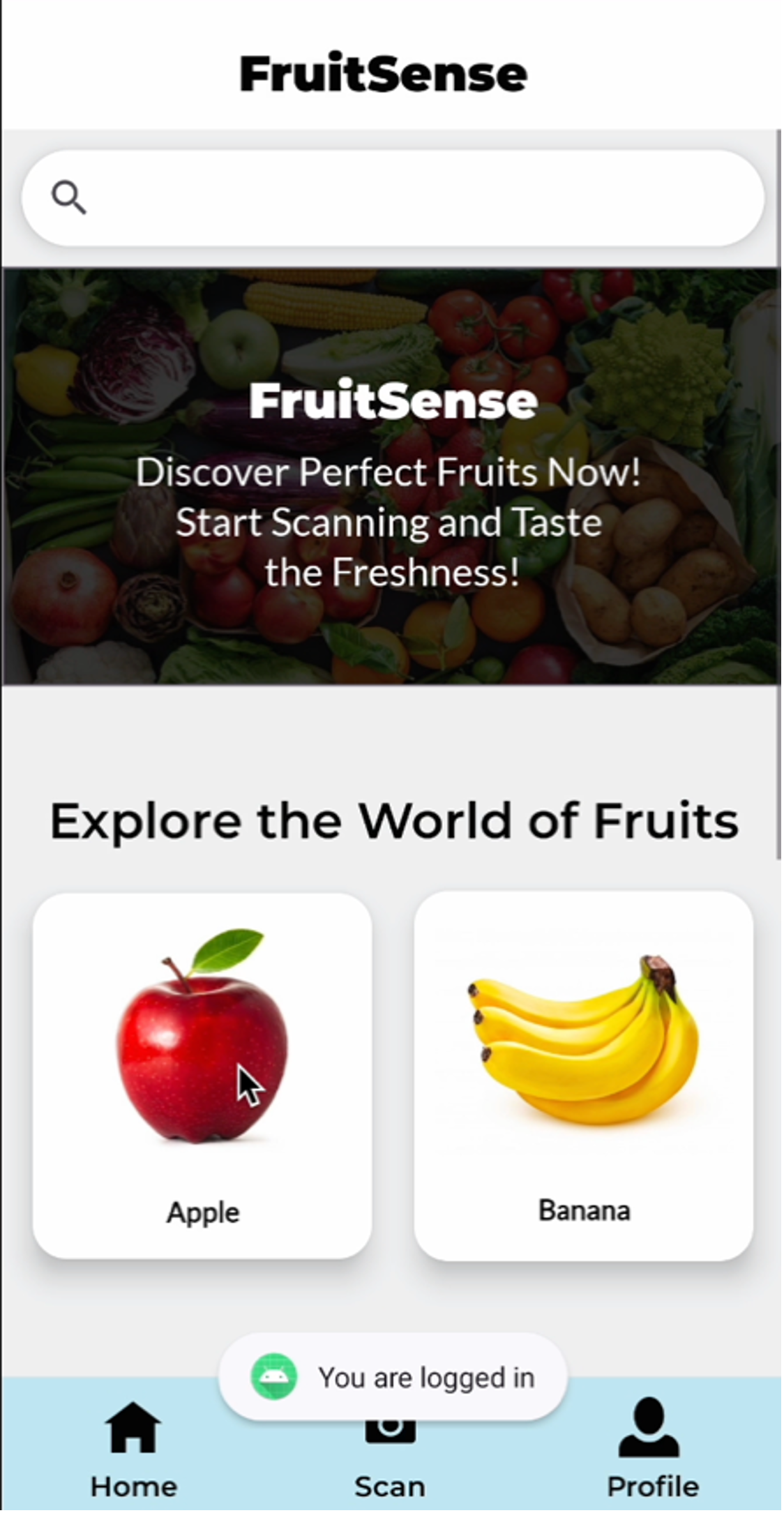
If users don’t have an account, they can click Sign-Up to create one. Just fill in the username, email, password, and phone number, hit Sign Up, and then head back to the sign-in page to log in which then redirect to the home page.
2. Home
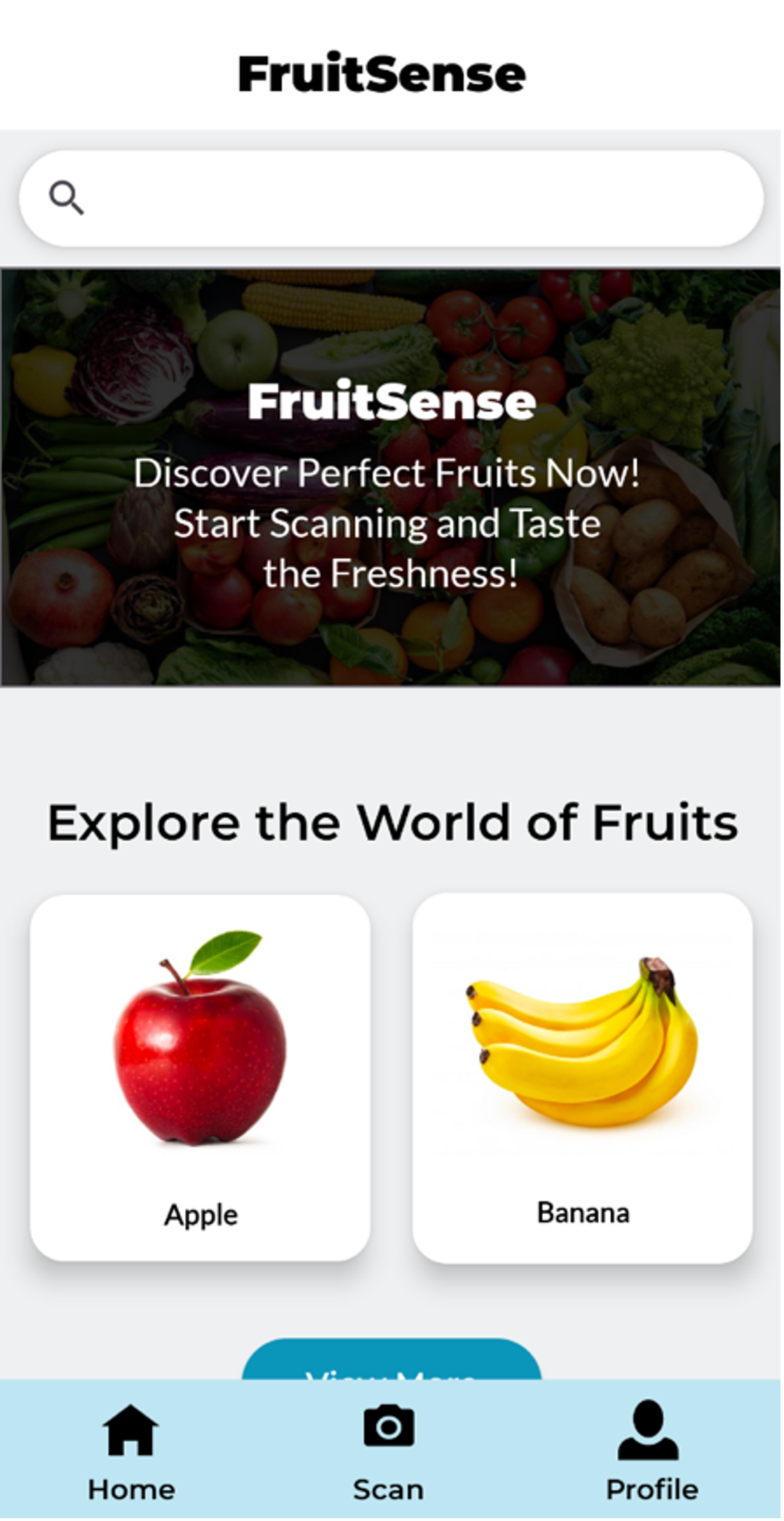
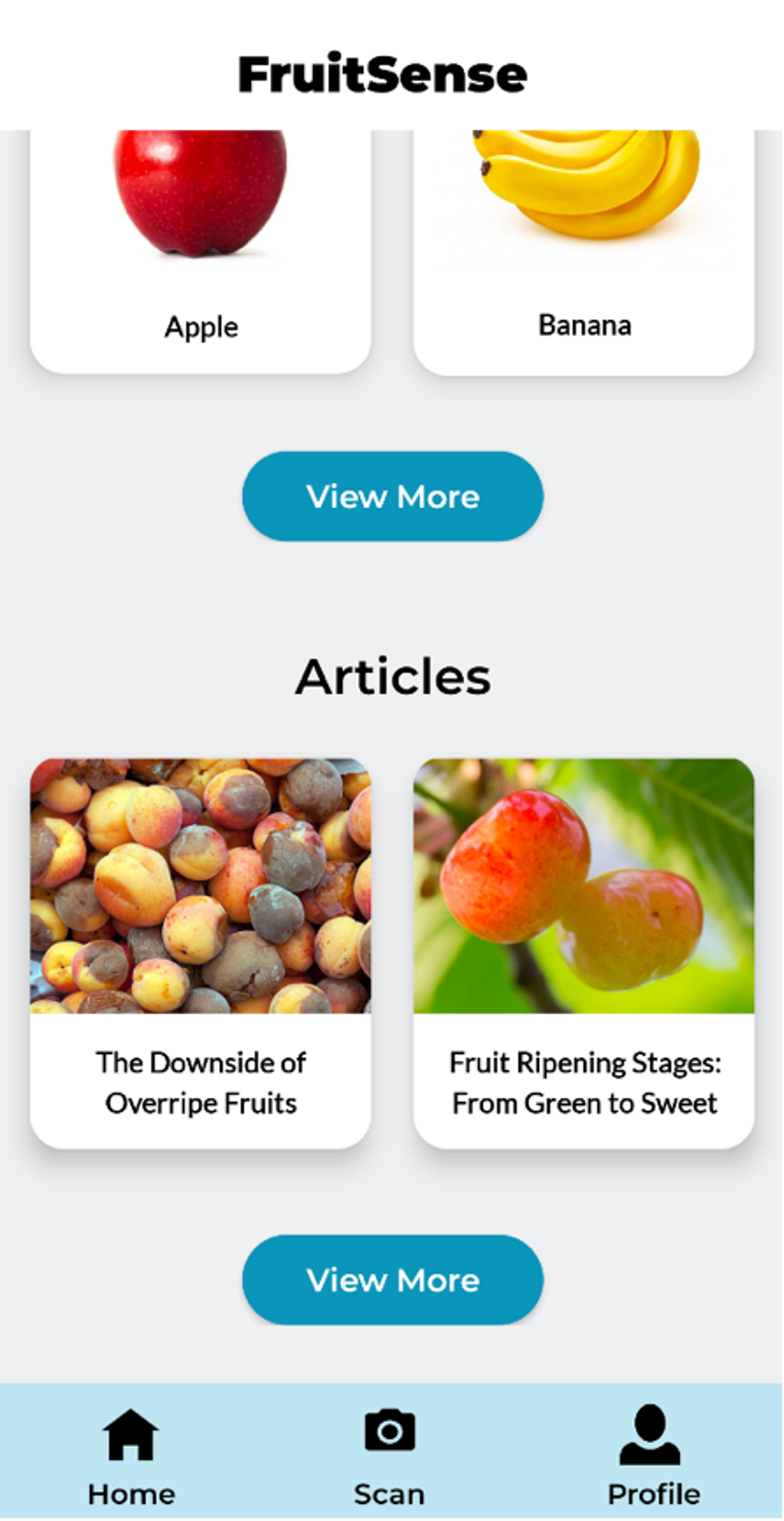
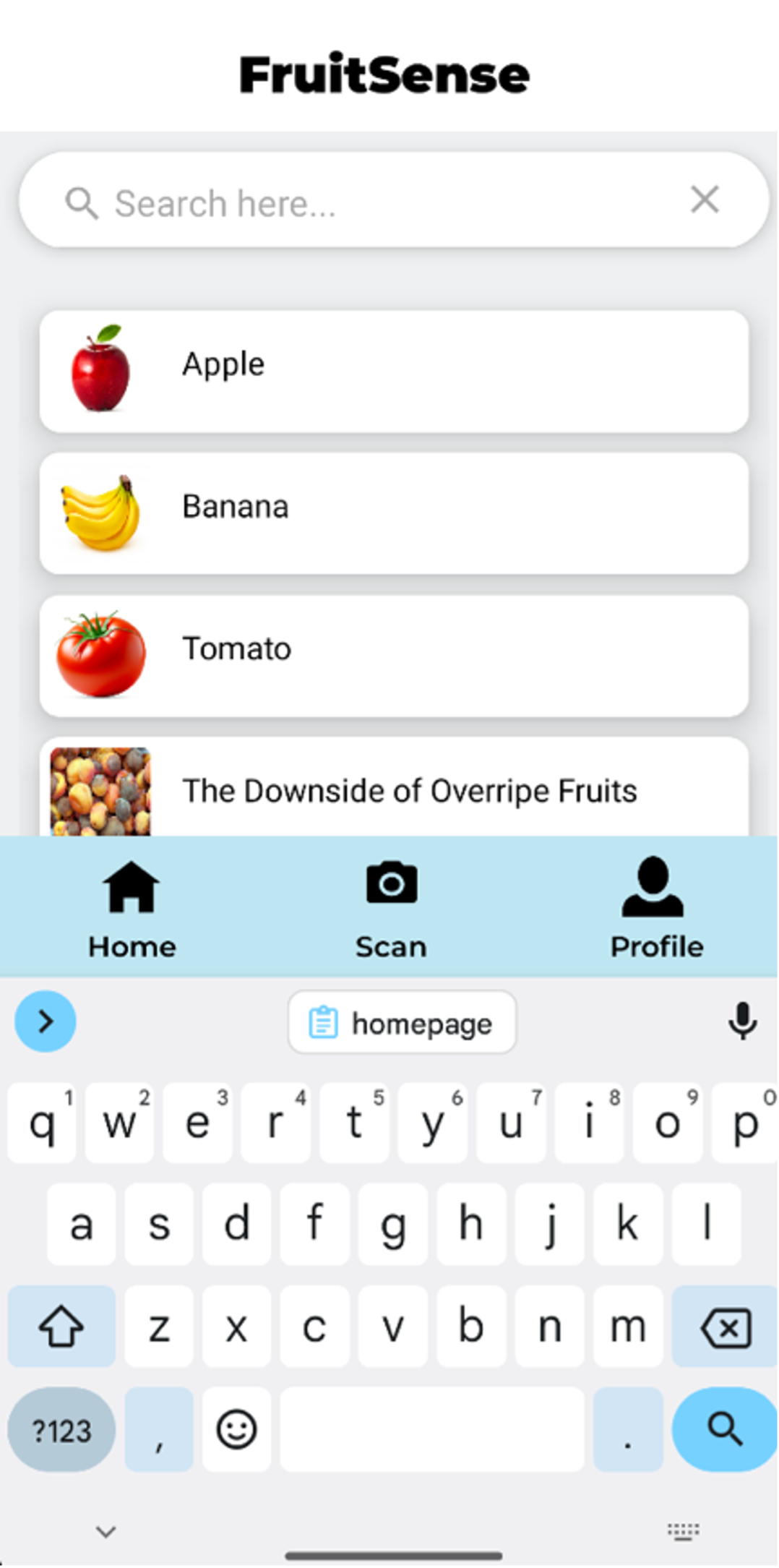
3. Fruit and Article
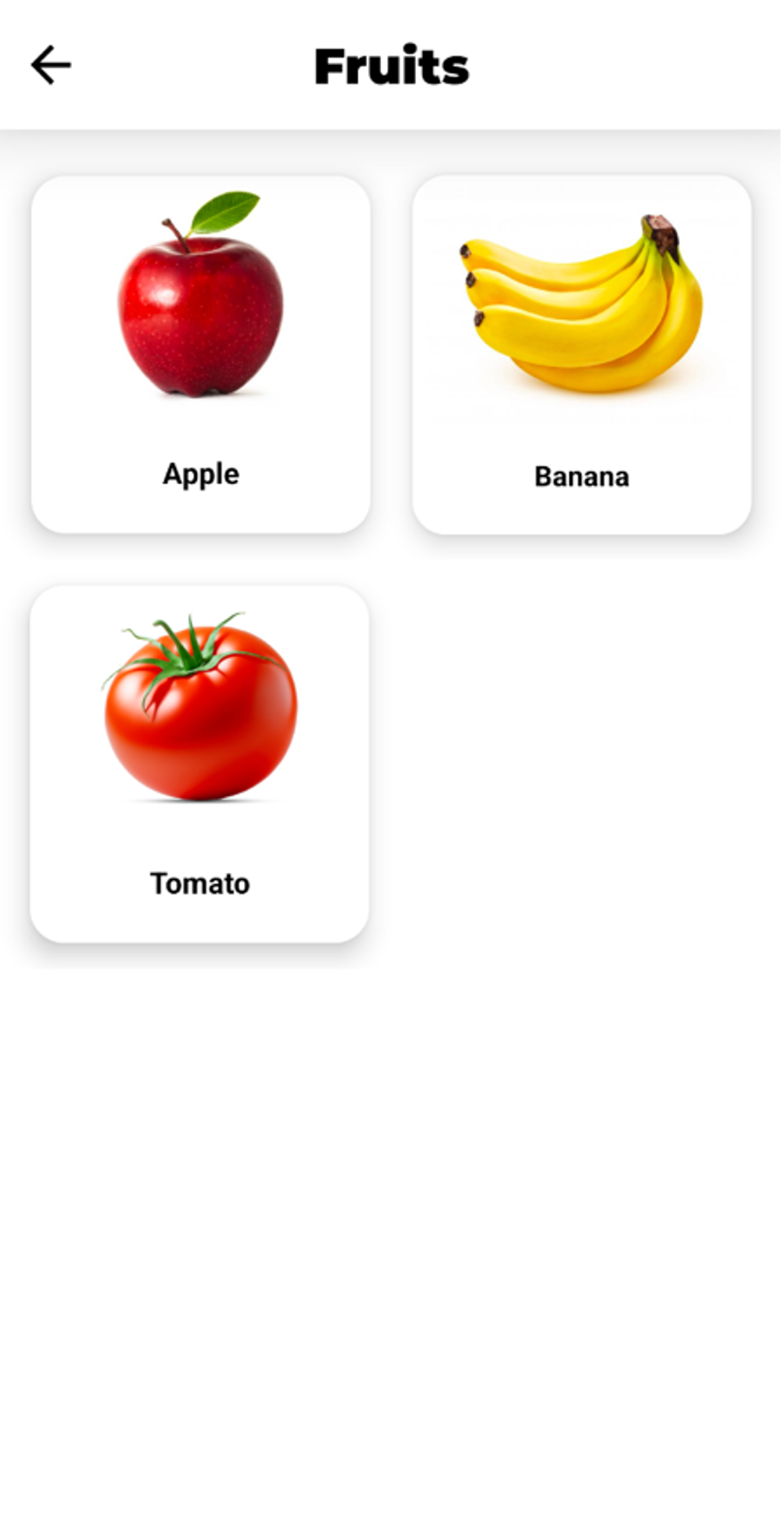
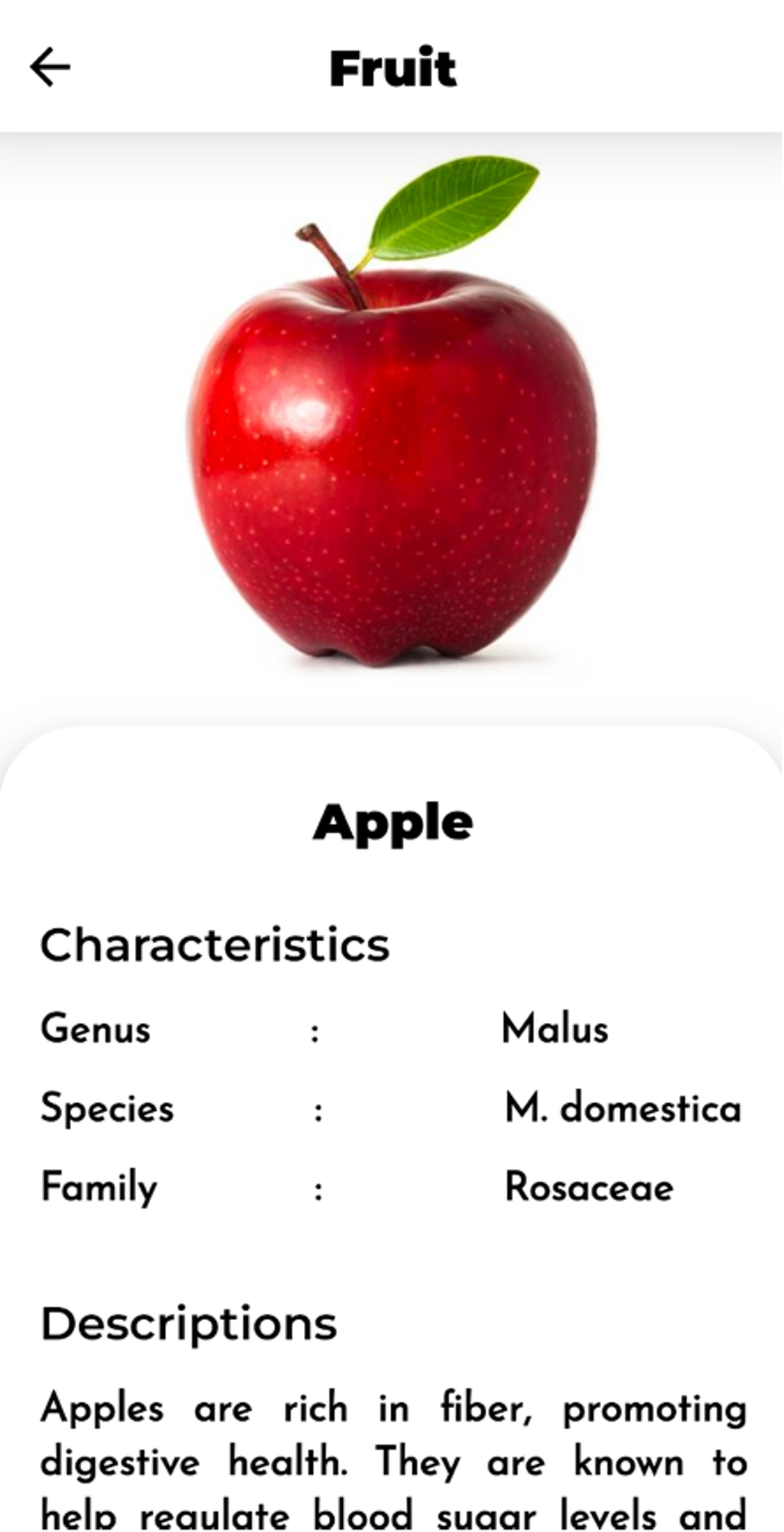
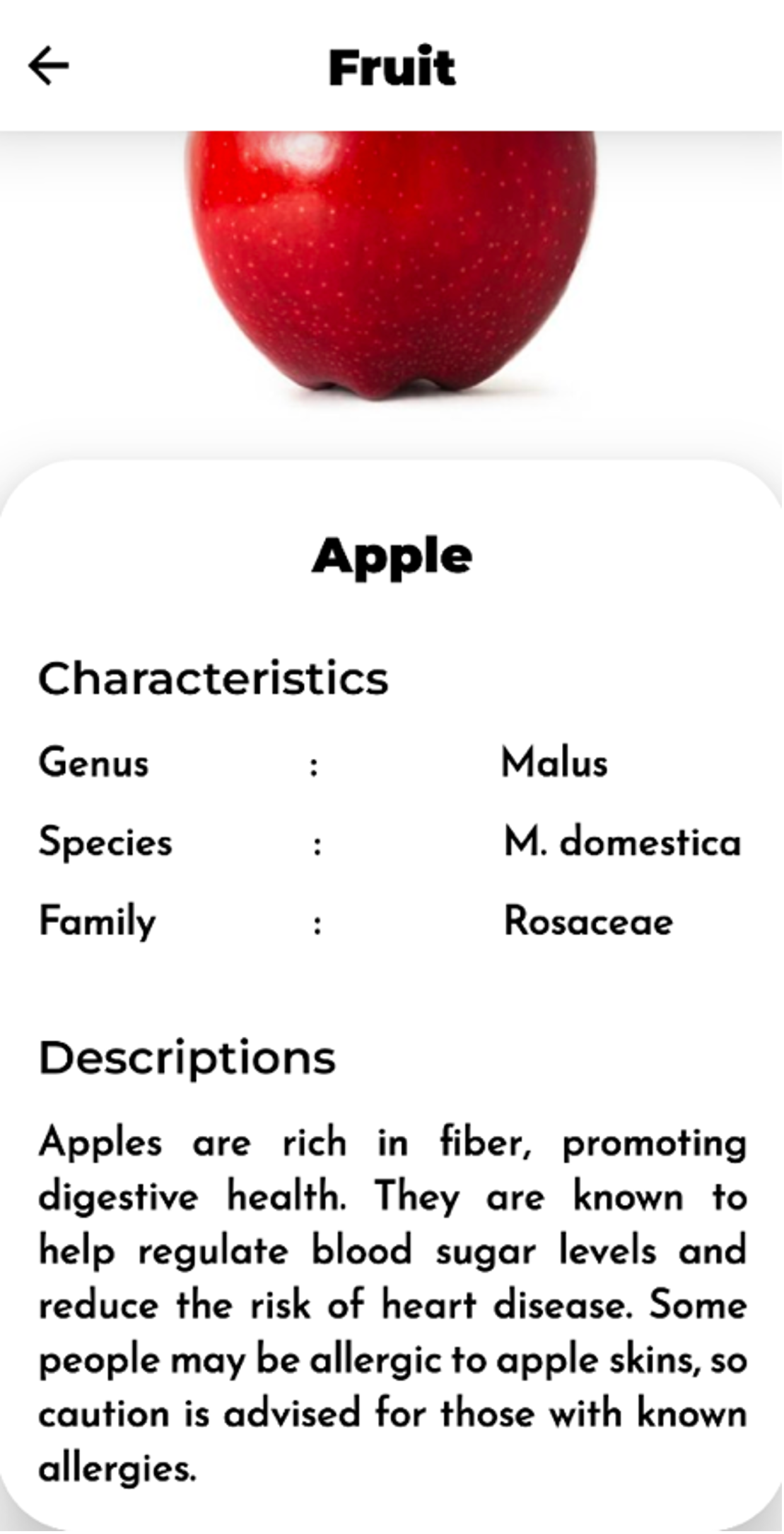
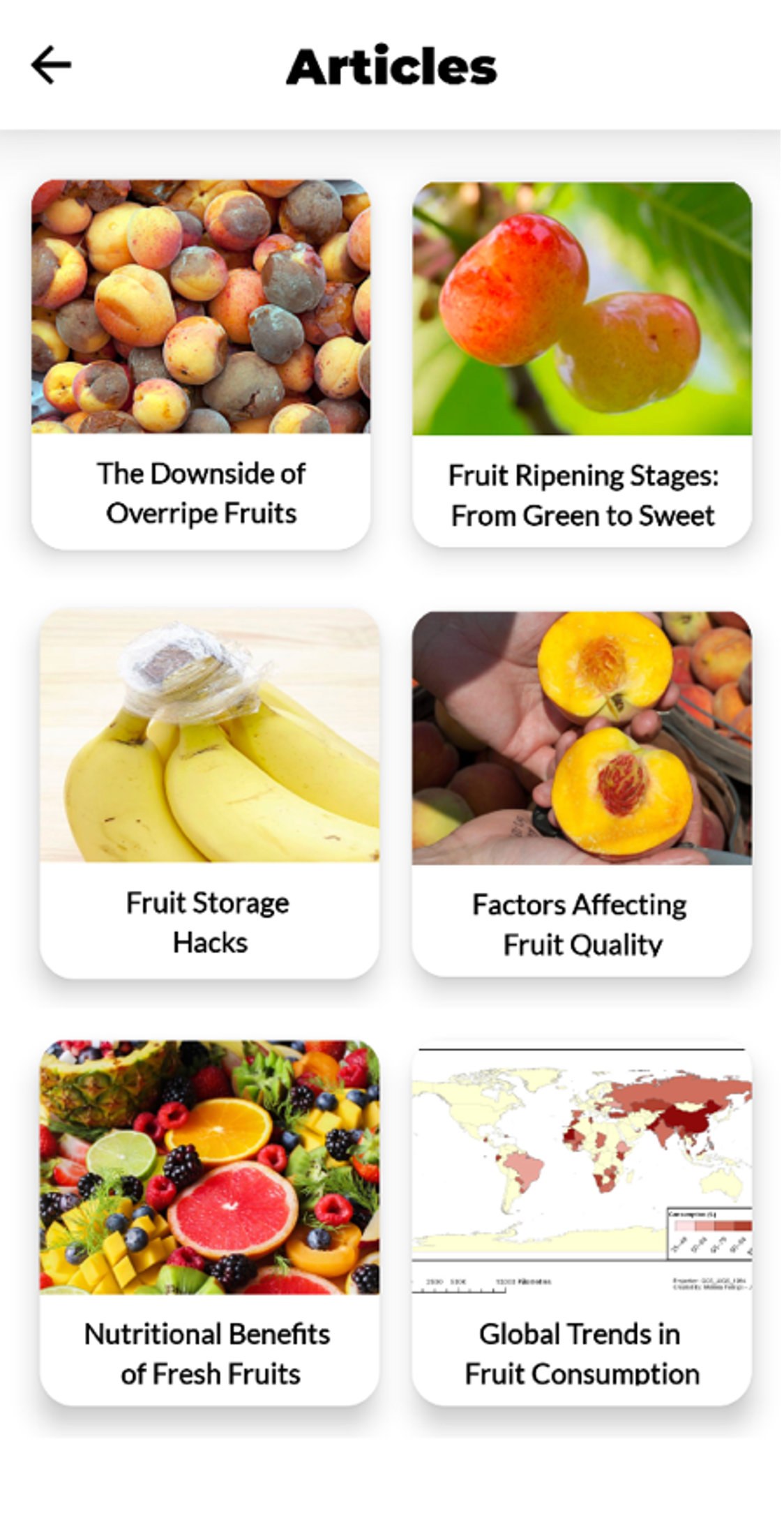
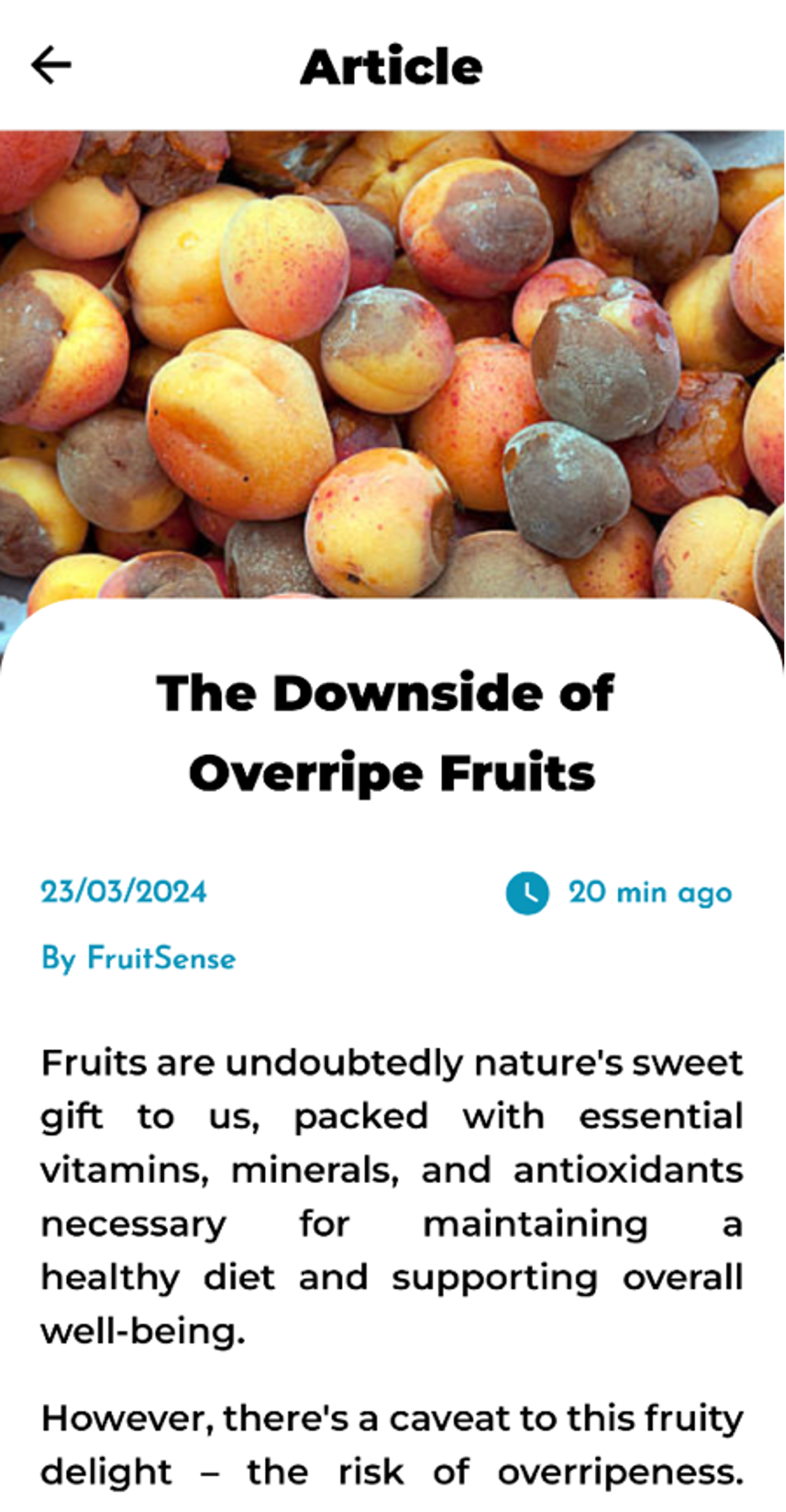
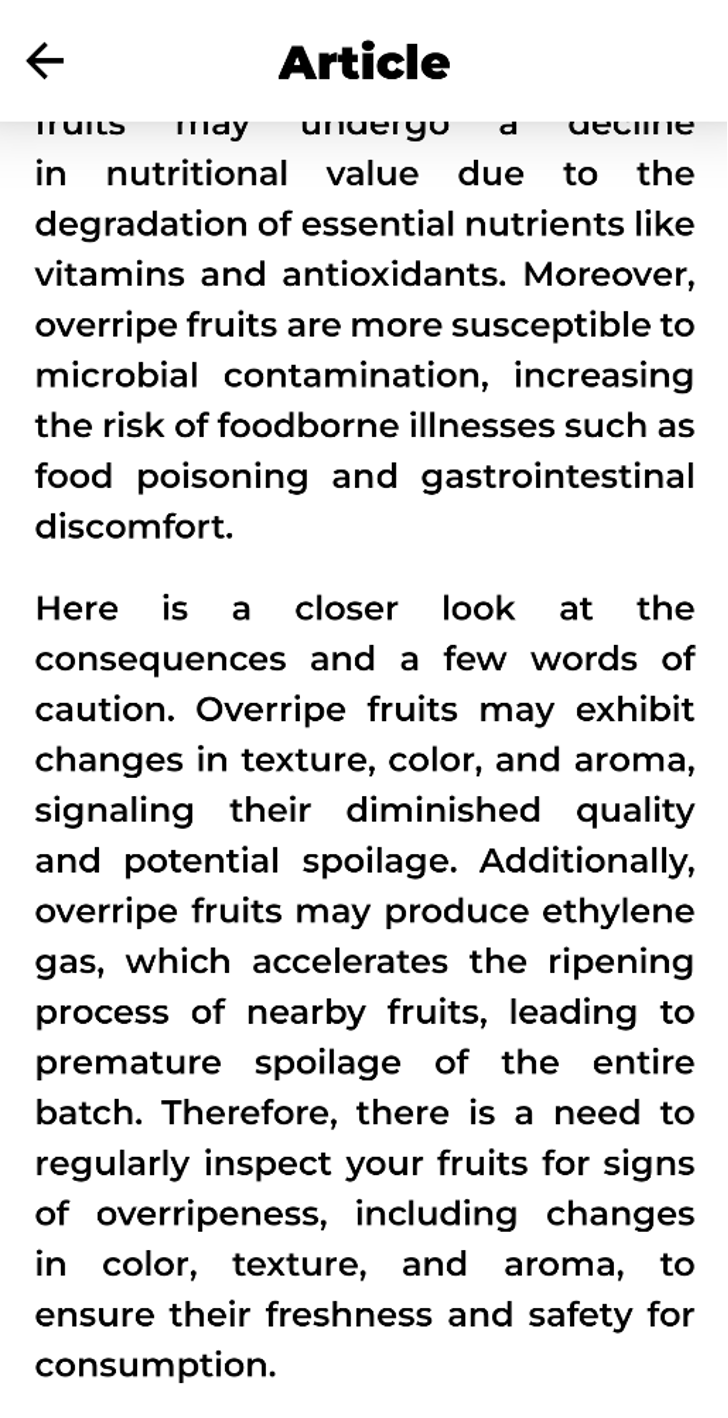
4. Scan
4.1 Scan Page
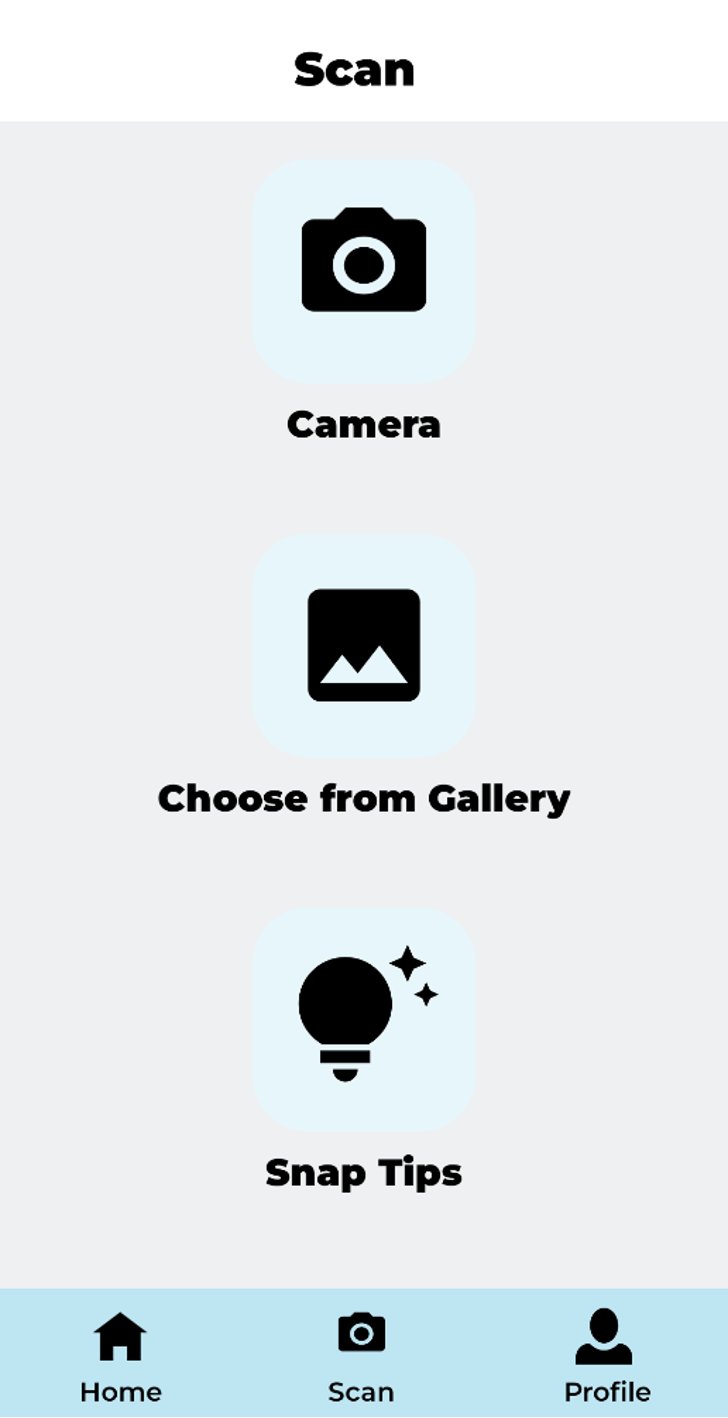
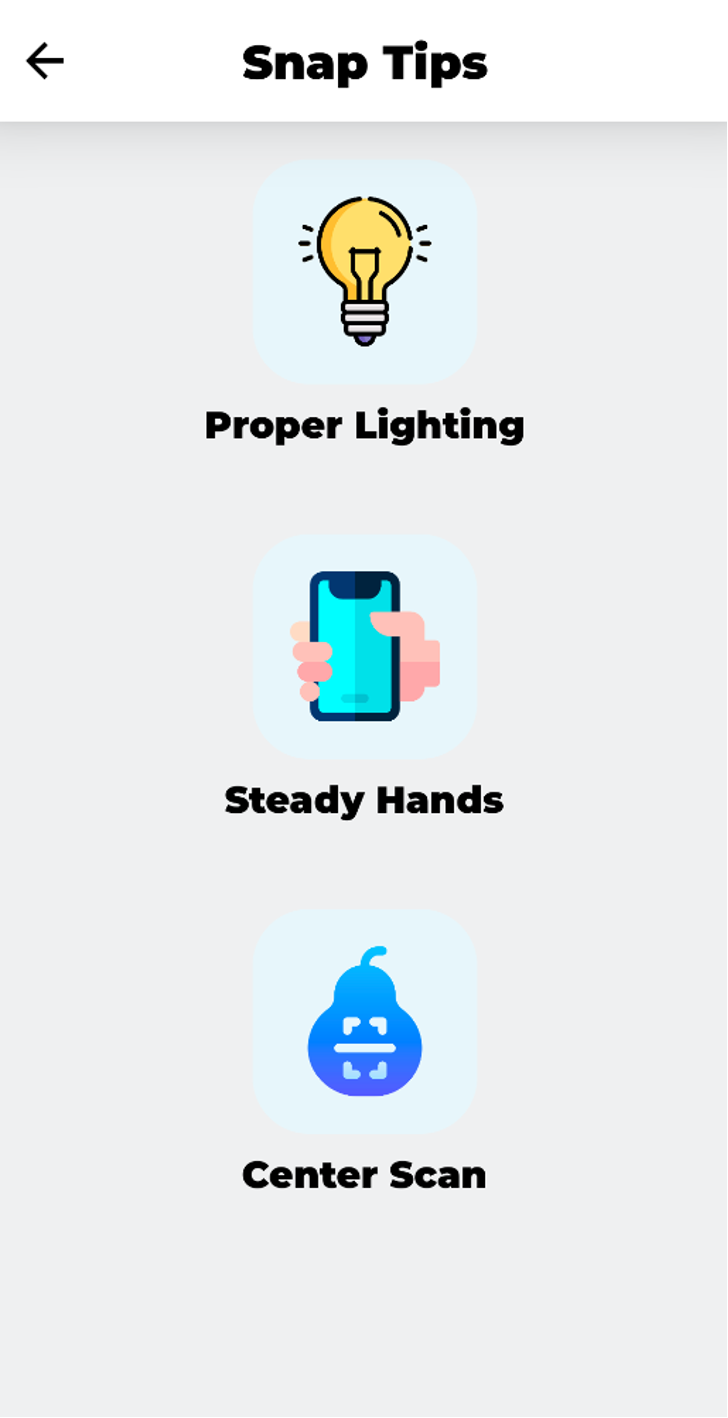
4.2 Scan Picture from Camera and Gallery along with Result Page
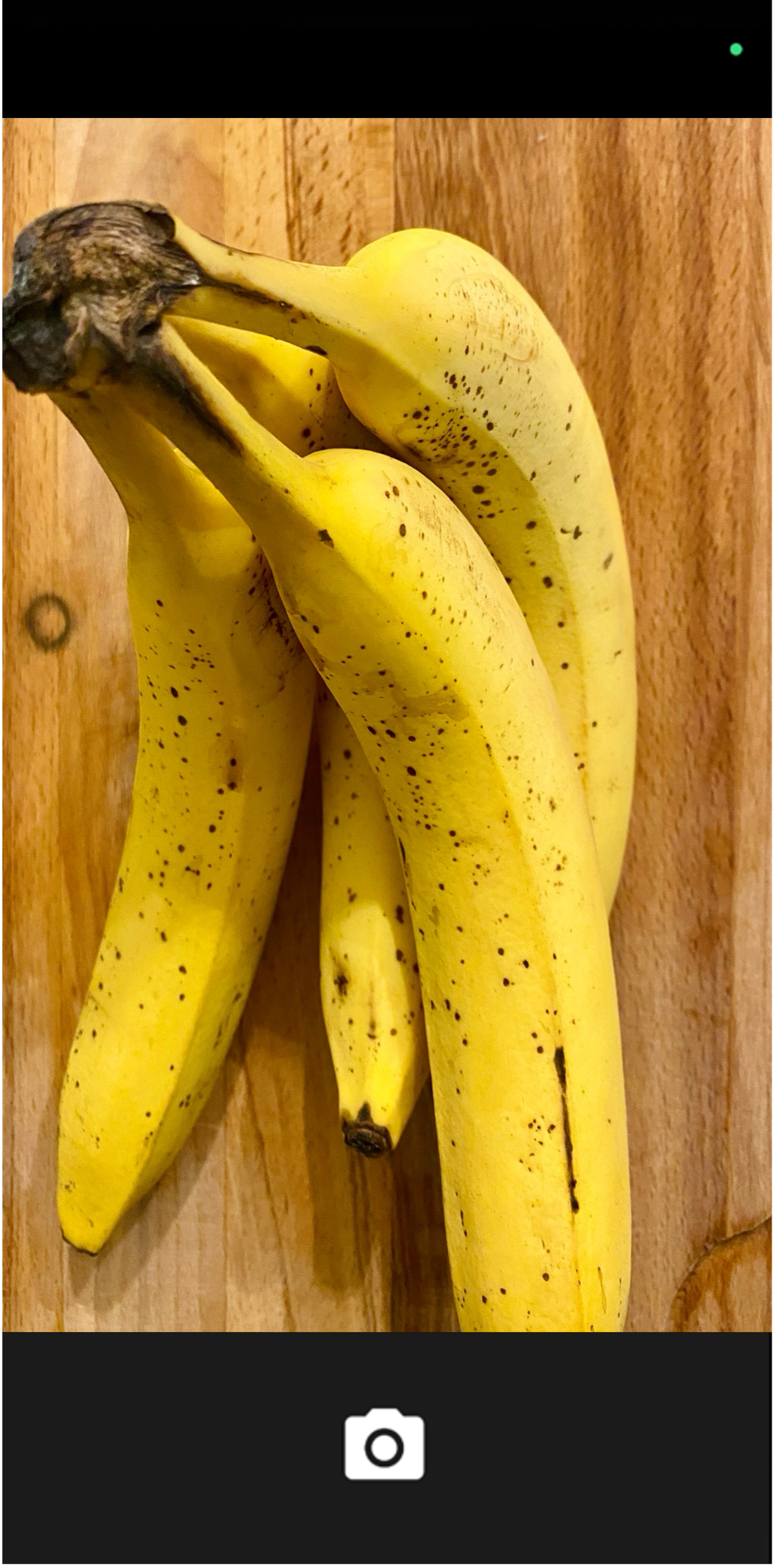
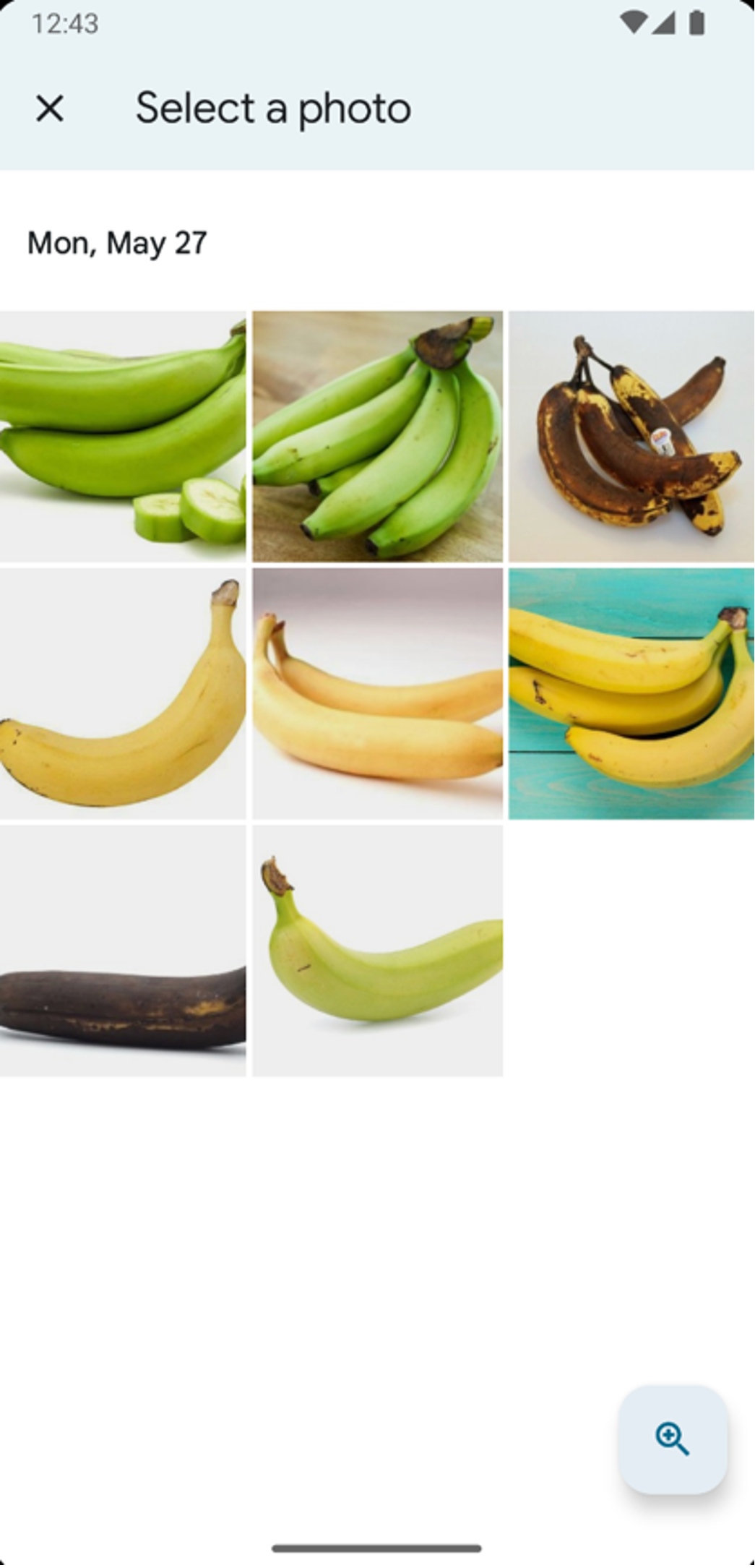
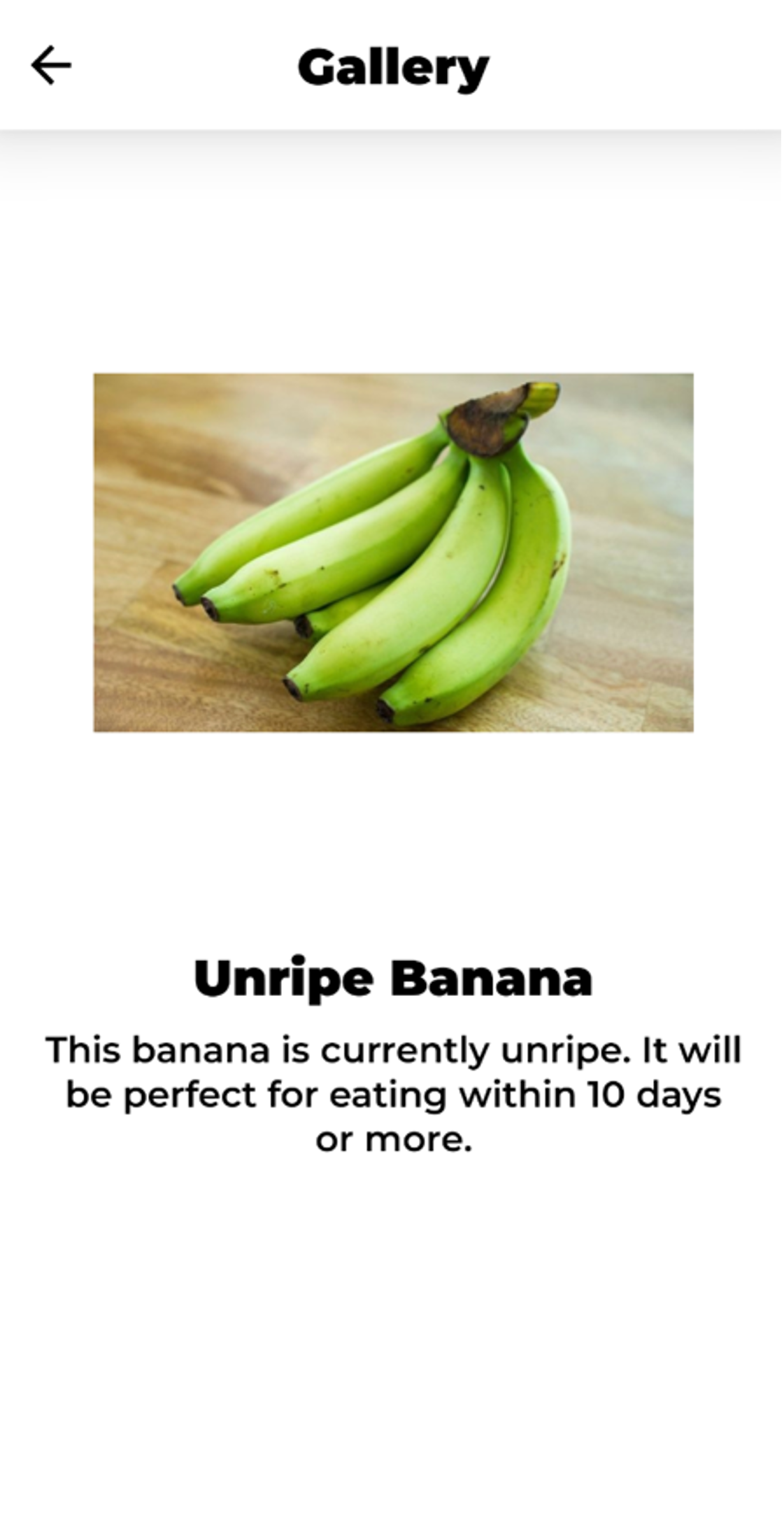
import org.tensorflow.lite.support.tensorbuffer.TensorBuffer;
private void classifyImage(Bitmap imageBitmap) {
Model model = Model.newInstance(getApplicationContext());
TensorBuffer inputFeature0 = TensorBuffer.createFixedSize(new int[]{1, 224, 224, 3}, DataType.FLOAT32);
ByteBuffer byteBuffer = ByteBuffer.allocateDirect(4 * imageSize * imageSize * 3);
byteBuffer.order(ByteOrder.nativeOrder());
Model.Outputs outputs = model.process(inputFeature0);
TensorBuffer outputFeature0 = outputs.getOutputFeature0AsTensorBuffer();
float[] confidences = outputFeature0.getFloatArray();
int maxPos = 0;
float maxConfidence = 0;
for (int i = 0; i < confidences.length; i++) {
if (confidences[i] > maxConfidence) {
maxConfidence = confidences[i];
maxPos = i;
}
}
model.close();
}
This block of code
resizes an image, prepares it for classification, feeds it into a machine learning model, and retrieves the output to determine the most likely class from and associated confidence score. The model is then closed to free up resources.5. Account
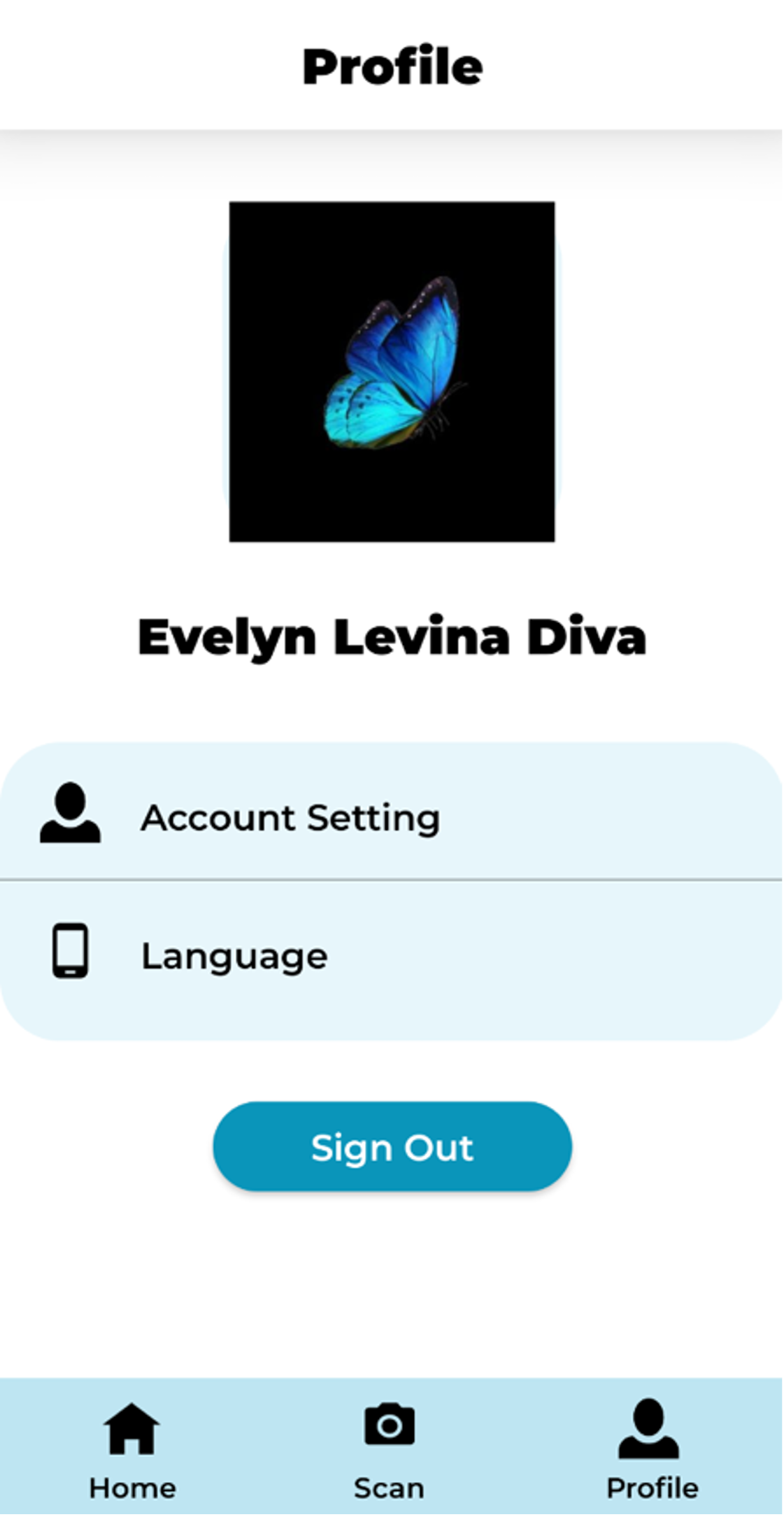
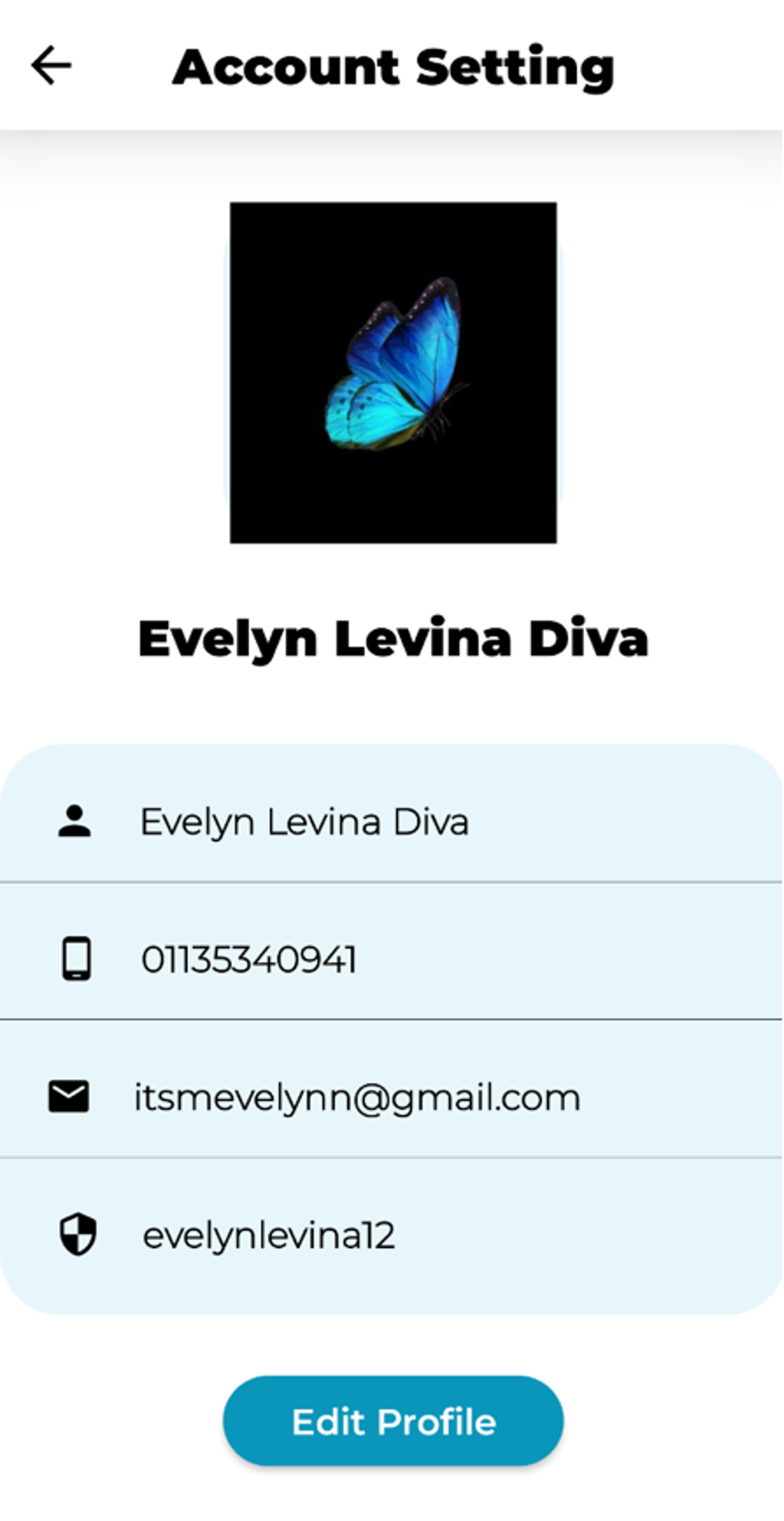
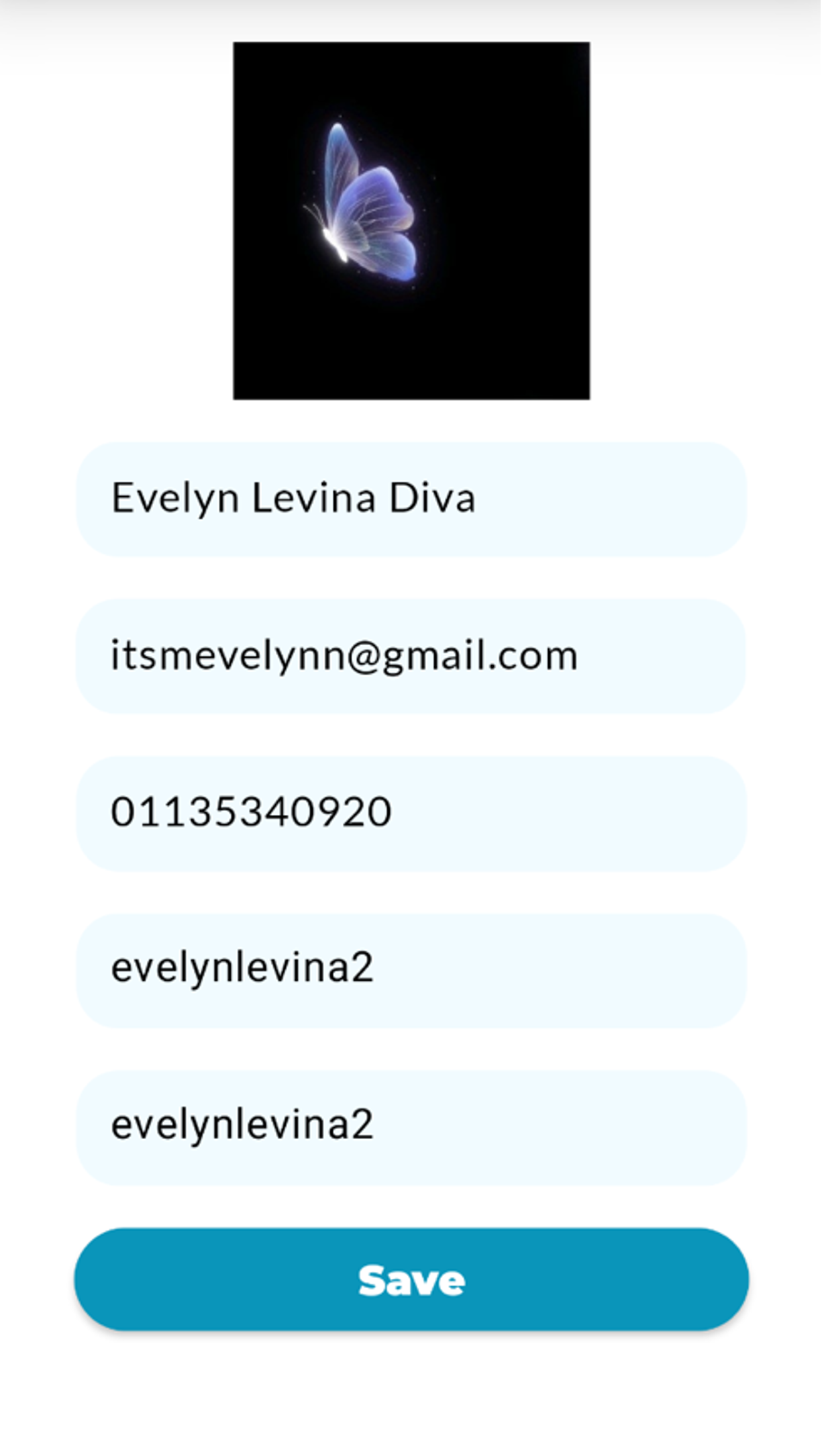
6. Switch Language
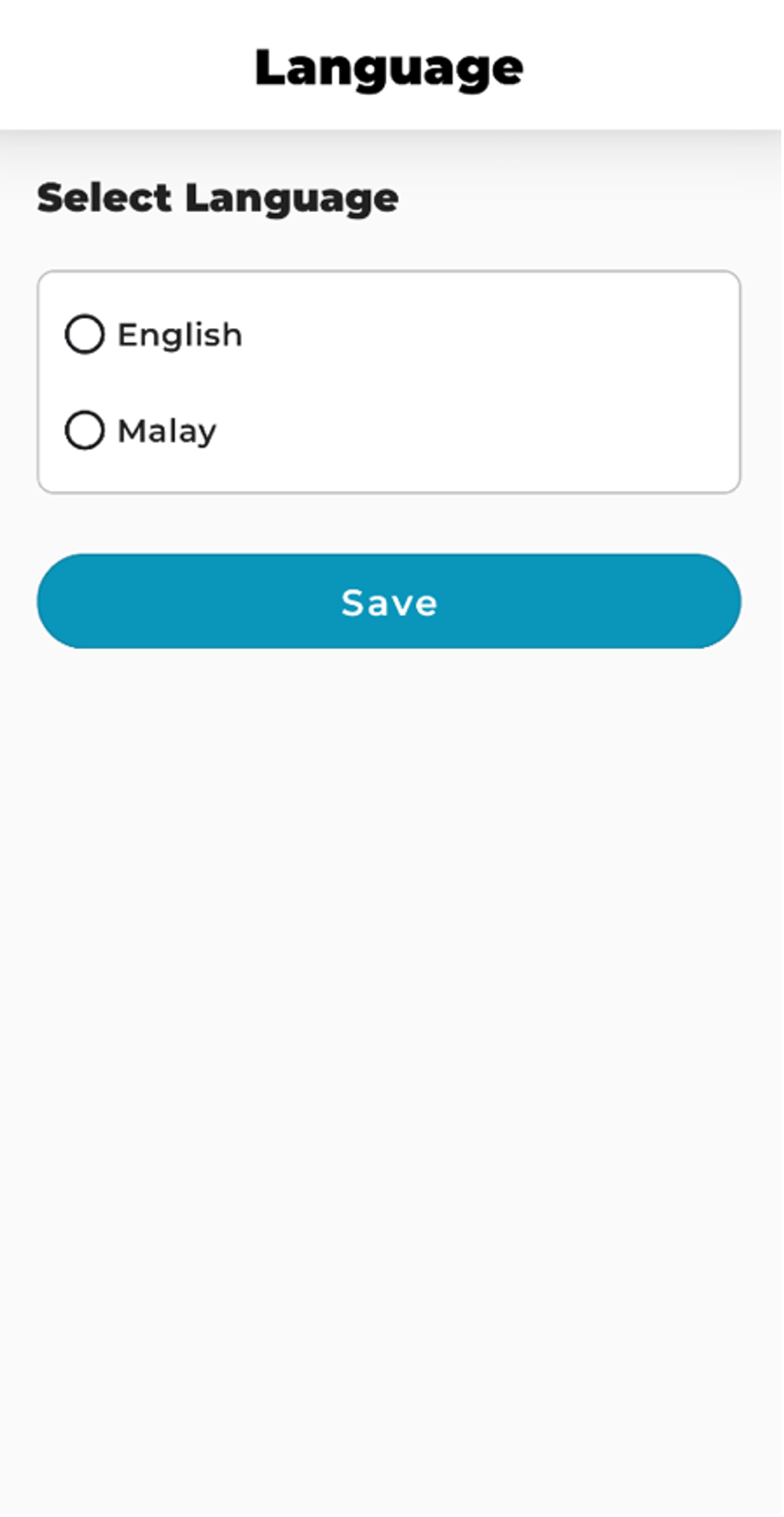
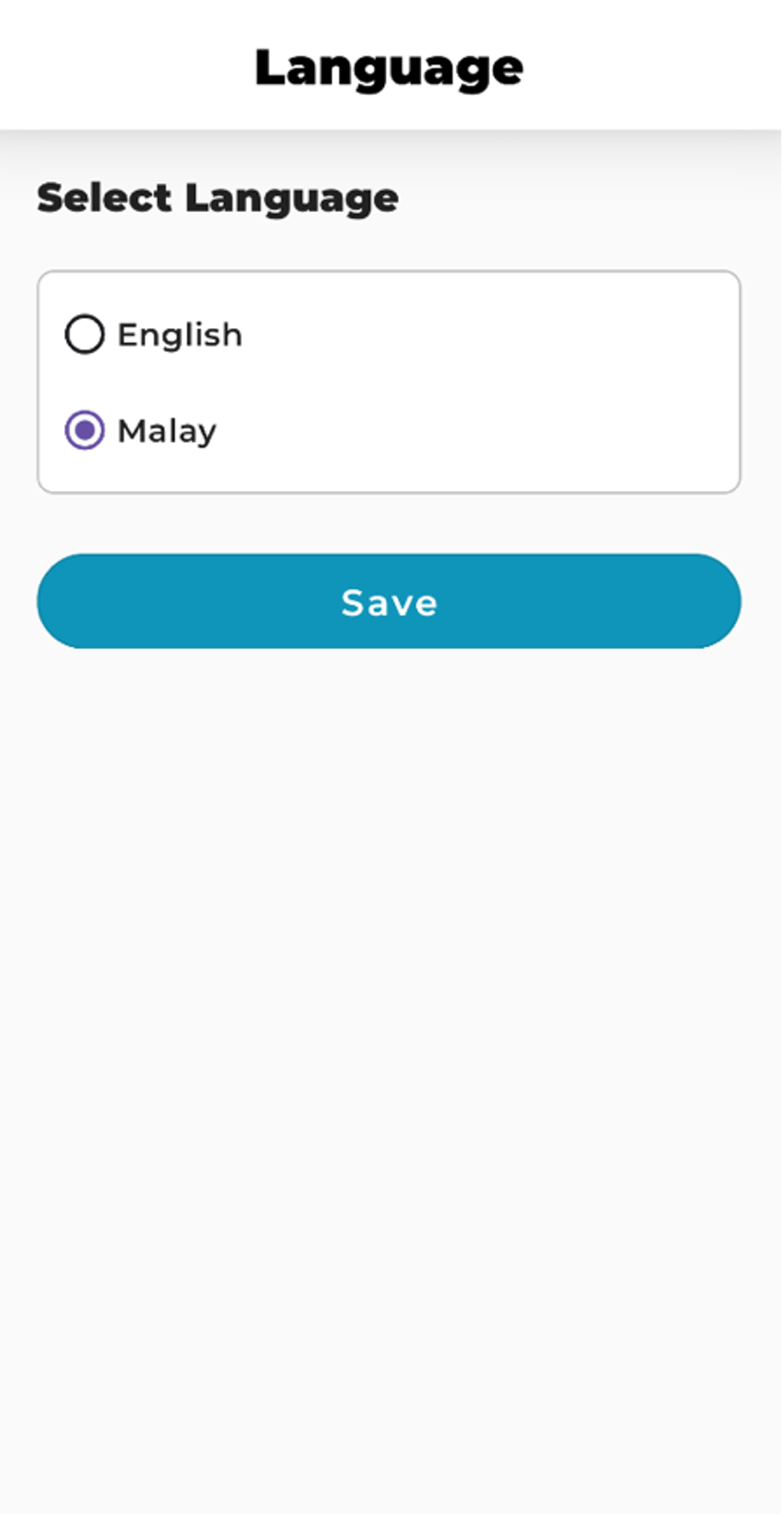
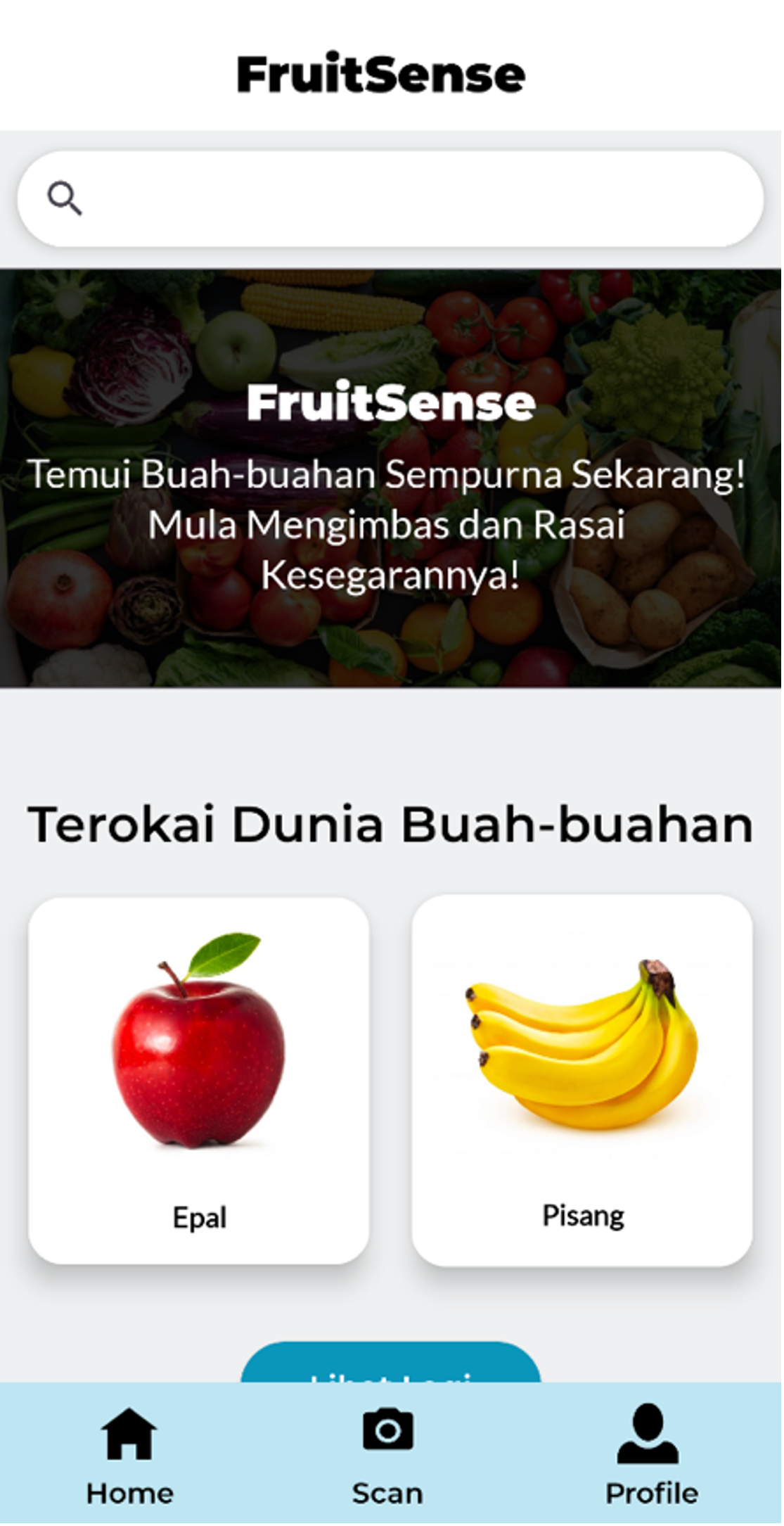
private void saveLanguageSelection() {
if (selectedRadioButton.getId() == R.id.radioButtonEnglish) {
languageCode = "en";
} else if (selectedRadioButton.getId() == R.id.radioButtonMalay) {
languageCode = "my";
}
SharedPreferences preferences = getSharedPreferences("AppPrefs", MODE_PRIVATE);
SharedPreferences.Editor editor = preferences.edit();
editor.putString("language", languageCode);
editor.apply();
// Apply language change
Locale locale = new Locale(languageCode);
Locale.setDefault(locale);
Configuration configuration = new Configuration();
configuration.setLocale(locale);
getResources().updateConfiguration(configuration, getBaseContext().getResources().getDisplayMetrics());
}
}
is used to save the selected language (in this case English or Malay) in SharedPreferences and redirect to the profile page.
Tech Stack
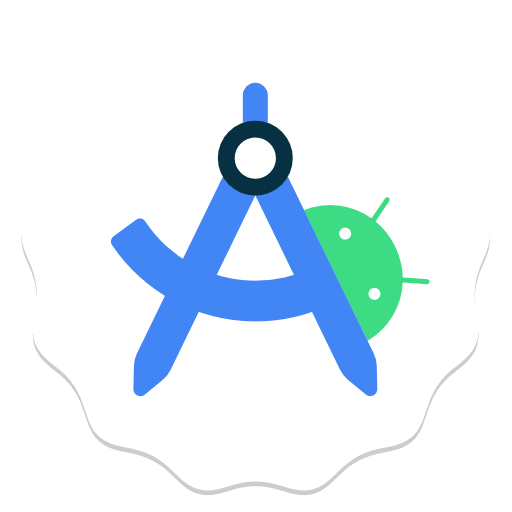
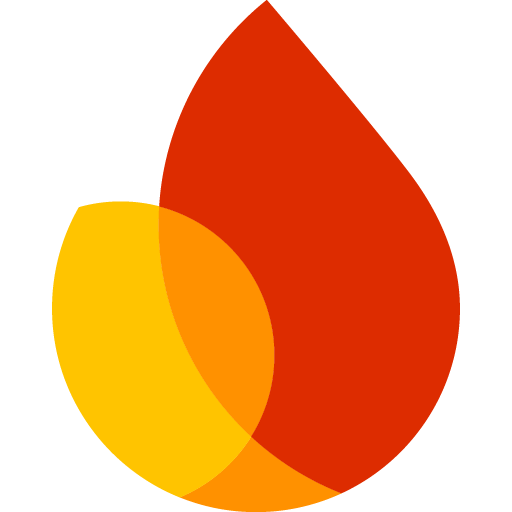
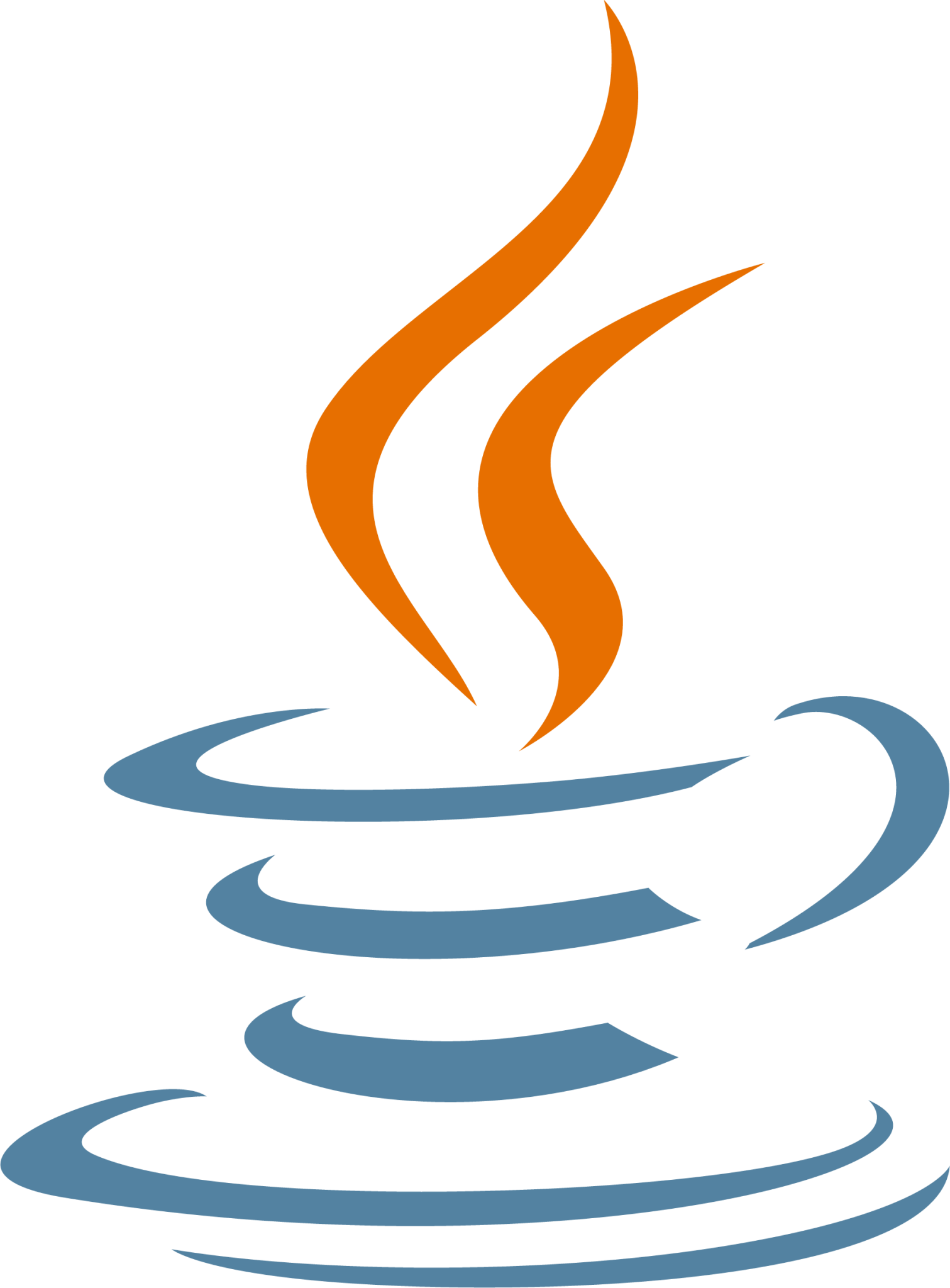
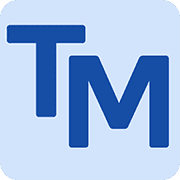
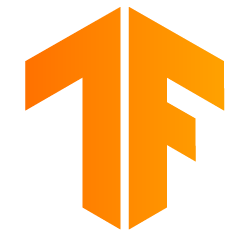